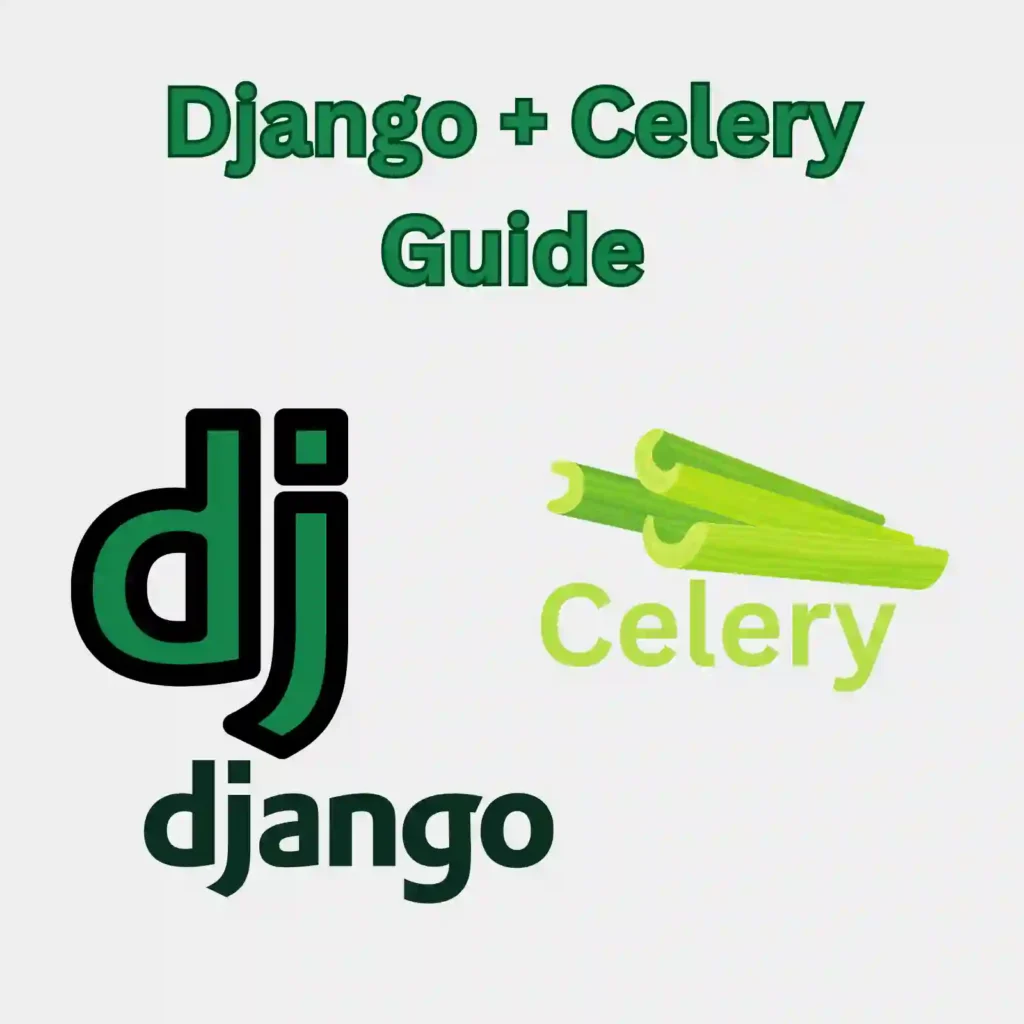
Dive into our comprehensive Django with Celery Complete Guide, designed to boost your web development skills by seamlessly integrating asynchronous tasks into your Django applications. Let’s dive deep into understanding the union of these two powerful technologies.
In Django, we have the power to build versatile and scalable web applications. Meanwhile, Celery provides us with an asynchronous task queue/job queue based on distributed message passing which indeed is focused on real-time operation.
Here’s how they interact together:
Components | Description |
---|---|
Django | This is a high-level Python web framework that encourages rapid development and clean, pragmatic design. |
Celery | A distributed task queue for Django. It gives you the ability to distribute work across threads or machines. |
RabbitMQ/Broker | This acts as a message broker between Django and Celery. Tasks (or messages) are published to the broker and then delivered to Celery workers to execute. |
Worker | The entity that performs computing operations in Celery. They pick up tasks from the queues and perform execution. |
Result Backend | This component is used to store task results. Although it’s optional, without it, your tasks will still run properly but the task results won’t be saved. |
Let me give informative light about the process of configuring Django with Celery to enhance your projects.
The first step is installing Celery using pip, our friendly package installer:
pip install celery
Make sure your Django project is created and configured adequately. Otherwise, create and navigate into your Django project before proceeding.
Next, we need to define Celery settings in the Django project. You can do so by creating a new module named
celery.py
. This file will contain such code:
from __future__ import absolute_import, unicode_literals import os from celery import Celery # set the default Django settings module for the 'celery' program. os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project.settings') app = Celery('your_project') app.config_from_object('django.conf:settings', namespace='CELERY')
Once done with configuration, it’s time to create some tasks to see this integration working. These tasks could fit inside any of your Django apps running within the project.
An example of a basic task may be a simple addition of two numbers and will look something like this:
from celery import shared_task @shared_task def add(x, y): return x + y
With all in place, we’ll just need to start the Celery worker:
celery -A your_project_name worker --loglevel=info
Django and Celery are teamed up now and ready to show their magic. Expect a fantastic horsepower boost while performing tasks asynchronously. To learn more, check out the [Official Django-Celery docs]. Working smoothly with these two tools could open up endless possibilities for your web-based projects. Trust me; coding life gets better when you know how to use Django with Celery.Django with Celery is a powerful combination. Integrating these two can lead to highly efficient and scalable web applications. Django, as we know it, is a high-level Python web framework that enables rapid development and pragmatic, clean design of web applications. Celery, on the other hand, is an asynchronous task queue/job queue system based on distributed message passing.(source)
To integrate Django with Celery, these steps have to be followed:
– Install Django and Celery: This is rather straightforward, quick, and can be done via pip:
pip install django celery
– Add a ‘celery.py’ file in your project folder: Here, you’ll need to configure Celery for your application. You’ll also require a broker such as RabbitMQ or Redis.
# Project_Name/celery.py from __future__ import absolute_import import os from celery import Celery os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'Project_name.settings') app = Celery('Project_name') app.config_from_object('django.conf:settings', namespace='CELERY') app.autodiscover_tasks()
– Update ‘\_\_init\_\_.py’ file: This file needs to be updated so that it’ll load celery every time Django starts.
# Project_Name/__init__.py from __future__ import absolute_import, unicode_literals from .celery import app as celery_app __all__ = ['celery_app']
– Creating tasks: With Celery successfully integrated and running, you can now define tasks within your Django app using @task decorator.
# apps/tasks.py from __future__ import absolute_import, unicode_literals from celery.decorators import task @task(name="sum_two_numbers") def add(x, y): return x + y
– Running workers: To run Celery worker which processes tasks, use this command:
celery -A project_name worker --loglevel=info
Where needed, changes should be made aligning with current project requirements. From here, possible enhancements could involve scheduling repeating tasks or adding priority queues to set an execution order (source).
At times, developers like myself might wonder about synchronous vs. asynchronous tasks. They both play their pivotal role. For example, if your Django views had to do a heavyweight, time-consuming operation (like sending emails to your users), it’s much more efficient to delegate the task to Celery. The action would be executed “in the background”, asynchronously, allowing Django to immediately respond back before the operation has finished, boosting performance and user experience.
In another scenario, if a task holds utmost importance and its result is crucial for the next line of operation, having it performed synchronously would prevent unnecessary trouble.
Hence, integrating Django with Celery not only unlocks the potential to vastly improve the user experience but also helps maintaining responsiveness and scalability of your application. By understanding what tasks to run where, our web apps remain efficient, robust, and prompt.
This table summarizes the key points:
Topic | Key Points |
---|---|
Django | A high-level Python web framework that encourages rapid development. |
Celery | An asynchronous task queue/job queue based on distributed message passing. |
RabbitMQ/Redis | Brokers required for configuring Celery. |
Synchronous VS Asynchronous | Know when to use which allows better performance & resource utilization. |
Finally, successful integration of Django with Celery does not mark the end but the beginning of many possibilities. Addition of features like retry in case of task failure, managing states of tasks, and implementing result backends (to monitor or inspect task statuses) are among subsequent steps that aim at improving efficiency and effectiveness of overall application. A multitude of intricate settings that Celery provides enhances further the range of opportunities, making every Django-Celery Integration unique and tailored towards optimized application performance.(source)
When we talk about developing web applications, the importance of asynchronously executing tasks cannot be understated. One tool that rises to prominence in this area is Celery, which when coupled with Django, a high-level Python Web framework, can dramatically enhance your application’s performance by offloading potentially lengthy operations and running them in different processes or even different servers. Let me dissect the process below:
The Core Rationale behind using Django and Celery
- Improving User Experience: Asynchronous task execution improves the user experience by allowing the server to respond quickly instead of waiting for a slow operation to conclude.
- Efficient Resource Utilization: Tasks can be scheduled on an ad-hoc basis, helping optimize resource utilization and thereby improving performance.
- Better Error Management: The implementation of retry policies helps manage errors effectively as tasks not executed successfully due to any reason are attempted again.
Integrating Django with Celery
To integrate Celery with Django, you’ll first need to install Celery using pip:
pip install celery
After installing Celery, you have to add it into your project. In your Django project, let’s create a new file named “celery.py” at the same level where “settings.py” lies:
from __future__ import absolute_import import os from celery import Celery from django.conf import settings os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project.settings') app = Celery('your_project') app.config_from_object('django.conf:settings') app.autodiscover_tasks (lambda: settings.INSTALLED_APPS)
This script creates an instance of the Celery library, configures it with your project settings and then autodiscovers tasks from all installed Django apps.
Django Views – Celery
Now, we’re going to make our Django view make use of one of these Celery tasks. Add/update your views.py file with the code:
from django.shortcuts import render from tasks import some_slow_operation def AsyncView(request): some_slow_operation.delay() return render(request, 'template.html', {})
In this code snippet, the asynchronous function ‘some_slow_operation’ is called with the ‘.delay()’ function which hands it off to Celery to perform when it’s able.
A Complete Guide to Running the Task Queue – Celery with RabbitMQ
Celery uses a message broker to pass messages between your application and Celery worker processes. You can use several message brokers like RabbitMQ, Redis, or Amazon SQS. Here, we’re using RabbitMQ.
To use RabbitMQ as a broker, make sure you’ve installed it first. After that, it’s necessary to configure Celery to use RabbitMQ:
app = Celery('my_celery_app', broker='amqp://guest@localhost//')
This tells Celery to transmit messages via AMQP to the local host where RabbitMQ presumably runs. You can now perform asynchronous tasks in Django with Celery!
Going Forward
By integrating Django and Celery, you’ve unlocked potential benefits of running async tasks, like enhancing user experience, using server resources efficiently or handling errors smartly, which goes a long way in developing robust applications.
Remember, this guide mainly aims to provide a concise introduction to using Celery with Django. Depending on the complexity of your application, there may be many nuances and situations to handle. For further reading, I highly recommend this excellent “First steps with Django” guide found on the official Celery documentation site.
The beauty of developing web applications with Django lies in its ability to automate many processes. Yet, while Django can handle most tasks seamlessly, some operations are computationally intensive or time-consuming and could potentially bring your application to a halt during execution.
This is where task queuing comes in. Task queuing in Django using Celery offers an efficient way to streamline processes, ensuring that you maintain fast, reliable application performance regardless of the heavy lifting going on behind the scenes.
What is Celery?
Celery is a robust, flexible distributed system for processing messages (which are essentially tasks) asynchronously by maintaining a queue where tasks are sent, then executed either immediately or at designed intervals.
Setting up Celery for Django
The process of integrating Celery with Django involves several steps. Here’s a comprehensive guide:
Installing and Configuring RabbitMQ
Start by installing RabbitMQ which serves as our message broker.
sudo apt-get install rabbitmq-server
After installation, enable and start the RabbitMQ service
sudo systemctl enable rabbitmq-server sudo systemctl start rabbitmq-server
Installing and Integrating Celery into a Django Project
Next, add Celery to your Django project. This is done by simply installing Celery via pip.
pip install celery
Then, create a
celery.py
module inside your main project directory. This file will contain the configuration for the Celery instance.
from __future__ import absolute_import, unicode_literals import os from celery import Celery os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project_name.settings') app = Celery('your_project_name') app.config_from_object('django.conf:settings', namespace='CELERY') app.autodiscover_tasks()
Creating and Running Tasks
In any of your Django apps, create a
tasks.py
file. Inside this file, define your tasks as functions then just decorate them with the @app.task decorator.
For instance:
@app.task def add(x, y): return x + y
You can run these tasks from elsewhere in your code like this:
from .tasks import add add.delay(4, 5)
The result is 9 but interestingly, the task would have been handled in the background.
To make sure our tasks get processed, we need to start a Celery worker from command line:
celery -A your_project_name worker --loglevel=info
Why use Django with Celery?
There are numerous reasons why you should consider using Django with Celery. These include:
- Asynchronous execution: With Celery, tasks don’t need to be run on the server synchronously. They are sent to the queue, processed and then results returned when they’re ready.
- Improved efficiency: Thanks to parallel execution, complex tasks can be broken down and executed simultaneously, hence speeding up completion times.
- Reduced server load: By executing tasks in the background, Celery ensures that your server doesn’t get overwhelmed even when there are tons of requests.
- Scalability: Celery helpfully supports multiple workers, meaning more tasks can be processed concurrently as your application grows.
Using Django with Celery takes bit of effort to set up, but the resulting boost in performance and efficiency can be substantial. For deeper coverage on the topic, here’s an online resource Real Python’s Guide on Asynchronous Tasks with Django and Celery. It provides great additional insights and a detailed walk-through of setting up a new Celery project with Django.Certainly, I’d love to assist with that. Let’s do a deep dive into common issues and their troubleshooting tips when working with Django together with Celery.
One of the most common challenges is ‘Task is not Executing’. This occurs when you have sent a task but it doesn’t seem like it’s going to be executed.
Typically, this might be due to:
-
- Celery worker process not running.
- Failure in task routing.
- A misconfiguration in your Django settings.
The first line of defense should be checking if your celery worker process is up and running. You could simply use command line to check status:
ps aux | grep celery
If no result comes out, it means your celery worker isn’t running. At this point, you’ll have to start it manually:
celery -A proj worker --loglevel=info
If celery worker appears to be running and tasks are still not dispatched, inspect the task itself and ensure proper routing.
To quickly navigate deep into the issue, here’s another trick: run celery worker in verbose mode. That way, you’ll get more detailed logs which will help to determine where exactly things go wrong.
When tasks begin to backlog in queue without processing, it’s time to inspect Django settings for Celery. It is an important step to establish the correct broker URL (source).
Check for these common mistakes in the CELERY_BROKER_URL setting:
-
-
- Connection protocol mismatch where you used something different from what your broker requires. Typical protocols include: amqp (RabbitMQ), or redis:// (Redis)
- Wrong username or password.
- Inappropriate virtual host for RabbitMQ
- Incorrect port specification.
-
Be sure to correctly set your Django CELERY_BROKER_URL configuration like this:
CELERY_BROKER_URL = 'amqp://guest:guest@localhost//'
Another rampant challenge is ‘Inconsistent Task States’. When a state of a task remains in PENDING or STARTED even after successful execution, or when states are mismatched across workers, it’s vital to remember that task results are strictly a Django-Celery feature designed only to track status and results. Therefore, consult your codebase and ensure appropriate results backend has been set:
result_backend = db+mysql://scott:tiger@localhost/foo
Finally, let’s tackle ‘Not Receiving Tasks’. Imagine a situation where everything seems in place – celery workers running, no backlog in queues… In this instance:
-
-
- Turn on visibility to unacked messages in RabbitMQ management plugin. Then inspect if any blocked connections exist.
- Ensure to properly kickoff tasks using ‘.delay()’ method or use ‘apply_async()’. An overlooked implementation detail often lies in here.
-
Happy coding with Django-Celery integration! This elucidation takes us through several key areas – an indispensible reference for anyone exploring the use of distributed task queues in Django applications. Not only does it address common challenges, but also arms you with approaches for efficient problem-solving, ensuring a smooth journey as you delve into Celery work processes. Check here for a complete Django with Celery guide!Incorporating Celery into a Django project is an excellent method to improve the performance of your web applications. There are a few advanced techniques you can use in scaling with Django and Celery which I’ll discuss here. All these methods are crucial, useful, practical, and highly recommended as a complete guide for Django with Celery.
1. Installing Celery and RabbitMQ
Firstly, start off by having Django, RabbitMQ, and Celery installed in your system. RabbitMQ works as a message broker for Celery. Install RabbitMQ by downloading it from their official website. After everything is installed, you can integrate Celery into your project by adding it in the apps of your settings.py file and configuring your RabbitMQ. Here’s a snippet of how you can do these:
INSTALLED_APPS = [ ... 'celery' ]
CELERY_BROKER_URL = 'amqp://your_rabbitmq_user:password@localhost:5672/your_virtual_host`
After setting everything up, run and check your worker. If things have been installed correctly, you’ll see the following after running the celery -A project worker –loglevel=info command:
[config] .> app: your_project:0x10fc87f98 .> transport: amqp://guest:**@localhost:5672// .> results: postgresql://guest:**@localhost/ .> concurrency: 4 (prefork) .> task events: OFF (enable -E to monitor tasks in this worker)
2. Offloading Tasks to Celery
The primary function of Celery is to take tasks that would drastically slow down your application’s user experience and to have them processed in the background while users continue browsing your site unaffected. By defining tasks in Celery, it takes the processing load away from your main thread. Here’s an example of how you can create a task:
from celery import shared_task @shared_task def my_background_task(): # task code here.
3. Building Scalability with the CELERYD_PREFETCH_MULTIPLIER
One major problem affecting large scale applications using Django with Celery is the issue of tasks being distributed unevenly across workers. This is often due to a configuration setting referred to as the CELERYD_PREFETCH_MULTIPLIER. If you have long-running tasks combined with quick tasks and you have many of them running together, setting the CELERYD_PREFETCH_MULTIPLIER to 1 ensures that tasks get evenly distributed across all workers and prevent bottlenecks in your application’s performance. Here’s an example of how you can set it:
CELERYD_PREFETCH_MULTIPLIER = 1
4. Using Flower to Monitor Your Tasks
Flower is a neat tool that provides real-time monitoring for Celery and can be plugged into your existing Django project easily. Visit the Flower documentation to learn more about installing and running it within your project.
These advanced techniques contribute to improving the performance of your web applications when scaling with Django and Celery. Ensure you follow best practices such as not using the database as a messaging system. Remember to test, experiment with different configurations, and adjust accordingly to suit your application’s needs.
Don’t miss official celery documentation on Django integration to explore more. Understanding how it fits together with django will help you troubleshoot issues that arise during development. And don’t forget the power and importance of always using the most recent versions, keeping your systems updated is key to efficient and trouble-free coding.When it comes to the seamless integration of asynchronous task management in Django applications, Celery has proven to be a dependable solution. The flexible and robust nature of this platform aids in executing numerous tasks at a go without obstructing the flow of the main program.
The power of Celery lies in its ability to navigate the complexities that result from managing queues of tasks, executing periodic tasks, and handling shared resources. Its event-driven architecture enables working with high volumes of messages seamlessly.
Here is an elementary example to show the basic steps to setup and use celery with Django:
#...project settings INSTALLED_APPS = ( ..., 'djcelery', ) BROKER_URL = 'redis://localhost:6379/0' #...then in your app import djcelery djcelery.setup_loader() @shared_task def add(x, y): return x + y
The entire guide aims at making it easier for developers to grasp how to manage tasks asynchronously using Celery with Django. The setup and configuration process, worker monitoring, handling shared tasks, setting up period tasks, working with Queues, and troubleshooting guides all contribute to the simplification of the overall understanding.
However, this is not an end but a beginning. There are yet much more advanced topics like chaining tasks, chunking, and other performance optimization techniques which one has to master as they dive deeper into it but this guide sets up any developer to kick start their journey.
When integrated correctly, there’s no denying the impact Celery can make on a Django application—improving user experience and helping scale services by efficiently managing system resources.
The guide offers more than just a basic introduction but a stepping stone towards mastering Django with Celery. So take a step forward and delve deep into the world of asynchronous programming with Django and Celery. This guide will remain your companion in that journey—happy coding!
For further reference, you could check the online documentation for Django with Celery. This resource provides extensive information and valuable insights into effectively using these tools together.
Hence, to perfect your skills in this area, follow this guide and practice implementing its teachings.