Introduction
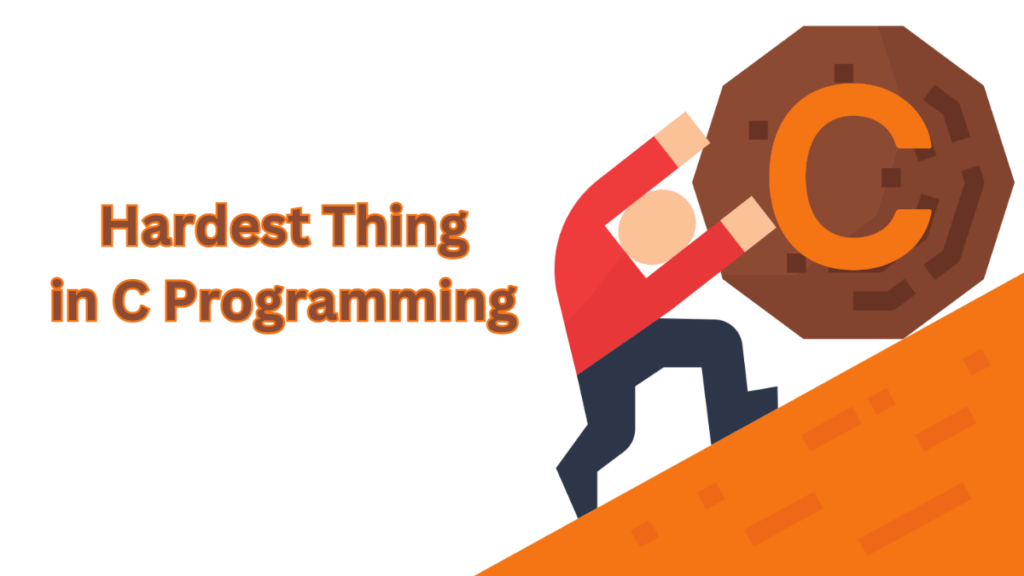
C was my first programming language. I have a love and hate relationship with it. Actually as the years pass by it’s more of a love relationship but that’s mainly because I don’t use it day to day like I used too in the past. Since I’m quite experienced I think I can have my fair say and give you my perspective on what I consider to be the most difficult thing with the C programming language.
Let me start by saying that there’s a plethora of things that you may find difficult and this really depends on which stage of your programming journey you are in. Back in the days for a lot of people C used to be the first language they’d learn. Since the most popular languages such as Python and Java weren’t really around you didn’t have many options but to work with it. Furthermore a lot of universities also teach the language as it’s good for fundamentals.
This however has shifted over the years and it’s no longer the case as Python and Java along with Javascript have dominated what you learn first and what you have to experience. This also means that C may not be your first language when you have to use it. In the case of the newcomers basically C is more of a thing they had to get into because otherwise they were using something else so I’m going to assume that you have some experience.
Memory Management
One of the biggest challenges in C is dealing with memory management. Because memory allocation and deallocation are manually controlled, it can become difficult to keep track of everything especially when working with large amounts of data. Here’s an example:
char *str = (char*) malloc(sizeof(char) * 100); if (!str) { printf("Memory could not be allocated."); } else { strcpy(str, "Hello World!"); printf("%s\n", str); free(str); }
This code allocates memory for a character string using the malloc() function, checks if allocation was successful, sets the value of the string to “Hello World!”, prints out the value, and then releases the memory using the free() function. If you forget to release the memory or attempt to access memory that has already been released, it can cause unexpected behavior or crash in your program.
Pointers and References
Pointers and references are both important concepts in the C language, but they can also be very confusing at first. Here’s an example:
int number = 42; int *pNumber = &number; printf("Value of number: %d\n", number); // 42 printf("Address of number: %p\n", &number); // Pointer/address printf("Value of pNumber: %p\n", pNumber); // Pointer/address printf("Value stored at pNumber: %d\n", *pNumber); // 42
In this code, we create a variable number and initialize it to 42. We also declare a pointer variable pNumber and point it to the address of number. We can then access the value of number using either its name or the pointer variable. The *pNumber syntax is used to dereference the pointer, which means it retrieves the value stored at the memory address pointed to by pNumber.
Function Pointers
Function pointers are another advanced feature in C, which can be difficult to work with. Here’s an example:
#include void multiply(int a, int b) { printf("%d x %d = %d\n", a, b, a * b); } void divide(int a, int b) { printf("%d / %d = %d\n", a, b, a / b); } int main() { int x = 10; int y = 5; void (*funcPtr)(int, int) = &multiply; funcPtr(x, y); funcPtr = ÷ funcPtr(x, y); return 0; }
In this code, we declare two functions named multiply and divide, which take two integers as input and print out the result of the operation. We then declare a function pointer called funcPtr that takes two integers as input and returns nothing. We set funcPtr to point to the multiply function, call it, then point it to the divide function and call it again. Function pointers are useful for passing functions as arguments to other functions or storing them in data structures.
Static Keyword
The static keyword in C can be a bit tricky to understand, but it has multiple uses. Here’s an example:
#include void update_counter() { static int counter = 0; printf("Counter: %d\n", ++counter); } int main() { for (int i = 0; i < 5; i++) { update_counter(); } return 0; }
In this code, we declare a function called update_counter that increments a static variable called counter each time it’s called. We then call this function five times from within a for loop. The static keyword applied to the counter variable ensures that the value of counter is retained between calls. Therefore, when we run this program, it will print out the values 1, 2, 3, 4, and 5.
Preprocessor Macros
Preprocessor macros are another feature of C that can be difficult to work with because they’re processed at compile-time. Here’s an example:
#include #define PI 3.14 int main() { double radius = 5.4; printf("Area of circle with radius %.2f: %.2f\n", radius, PI * (radius * radius)); return 0; }
In this code, we use a preprocessor macro to define the value of PI as 3.14. We then use it to calculate the area of a circle with radius 5.4 by multiplying it by the square of the radius. This code will output “Area of circle with radius 5.40: 91.56”.
File I/O
Working with files in C can be a bit complicated due to the relatively low-level nature of the I/O functions. Here’s an example:
#include int main() { FILE *fp; char ch; fp = fopen("example.txt", "r"); if (fp == NULL) { printf("File could not be opened."); } else { while ((ch = fgetc(fp)) != EOF) { printf("%c", ch); } fclose(fp); } return 0; }
In this code, we declare a file pointer called fp and a character variable called ch. We then use the fopen() function to open the file “example.txt” for reading, checking if it was successful. If it is, we use a while loop along with the fgetc() function to read each character from the file until we reach the end of file marker (EOF). We then print out each character, and finally close the file using fclose().
Recursion
Recursion, or calling a function inside itself repeatedly, can be both powerful and complex, especially in C programming. Here’s an example:
#include int factorial(int n) { if (n == 1) { return n; } else { return n * factorial(n - 1); } } int main() { int num = 5; printf("Factorial of %d: %d\n", num, factorial(num)); return 0; }
In this code, we define a recursive function called factorial that calculates the factorial of a number by calling itself with progressively smaller values until it reaches the base case of n = 1. Then we call this function on num which has a value of 5, leading to output “Factorial of 5: 120”.
Multidimensional Arrays
Declaring and manipulating multidimensional arrays can be challenging in C as you need to step through several dimensions in any loop. Here’s an example:
#include int main() { int test[3][2] = {{2,5},{3,6},{4,7}}; for(int i = 0; i < 3; ++i) { for(int j = 0; j < 2; ++j) { printf("%d ",test[i][j]); } printf("\n"); } return 0; }
In this code, we declare a two dimensional array and initialize its values. We have an outside loop that iterates through rows and an inner loop to iterate through all columns of the current row, finally printing them in a formatted manner.
Pointers to Functions
While working with function pointers can be challenging, sometimes you may also need to work with Pointers to Functions. Here’s an example:
#include int add (int x, int y) { return x+y; } int subtract (int x, int y) { return x-y; } int main () { int (*fun_ptr_arr[])(int, int) = {add, subtract}; int result1 = fun_ptr_arr[0](15, 10); int result2 = fun_ptr_arr[1](15, 10); printf("Addition Result: %d\n", result1); printf("Subtraction Result: %d\n", result2); return 0; }
This code declares an array of function pointers fun_ptr_arr which points to functions add and subtract. We then call both of these functions and store the results in result1 and result2, respectively. Finally, we print out both of the results to the console.
Dynamic Memory Allocation
Dynamic memory allocation is done when you want to allocate memory at run-time using functions like malloc or calloc. Here’s an example:
#include #include int main() { int* ptr; int n, i; printf("Enter number of elements: "); scanf("%d",&n); ptr = (int*) malloc(n * sizeof(int)); if(ptr == NULL) { printf("Insufficient Space.'); return 0; } for(i = 0; i < n; ++i) { printf("Enter element %d: ",i+1); scanf("%d", ptr + i); } printf("Array elements : \n"); for(i = 0; i < n; i++) { printf("%d\n",ptr[i]); } free(ptr); return 0; }
This code declares a pointer variable ptr, asks the user to enter the number of elements they want to input, and allocates memory accordingly. It executes a loop until each element is filled by taking inputs from the user, and then, finally prints out all elements in the array. Additionally, it also frees up memory allocated previously by calling free() on the pointer after printing the output.
Conclusion
By now you have probably come to a realization.. that C is unlike most high level programming languages due to it’s nature of giving you access to low level constraints. As you know with great power there’s also great responsibility. If you do not master those concepts properly it can come back biting you pretty hard.
Since I love C and I love the control it gives me I will not give it up for anything, especially when I want to achieve an application that’s running fast and is lightweight. However I understand that if you come more from a laid back point of view where you just want the language to handle your memory and do things for you C may not be the best language to cover what you need.
Let me close by saying that I believe anyone can become a master in C as long as they put the time and effort of understanding properly the fundamentals and is ready to take a deeper dive than they would if it was a simpler language like Python.
Related
- Why Stack Is Faster Than Heap
- Easy programming languages for beginners
- What Happens When Stack and Heap Collide