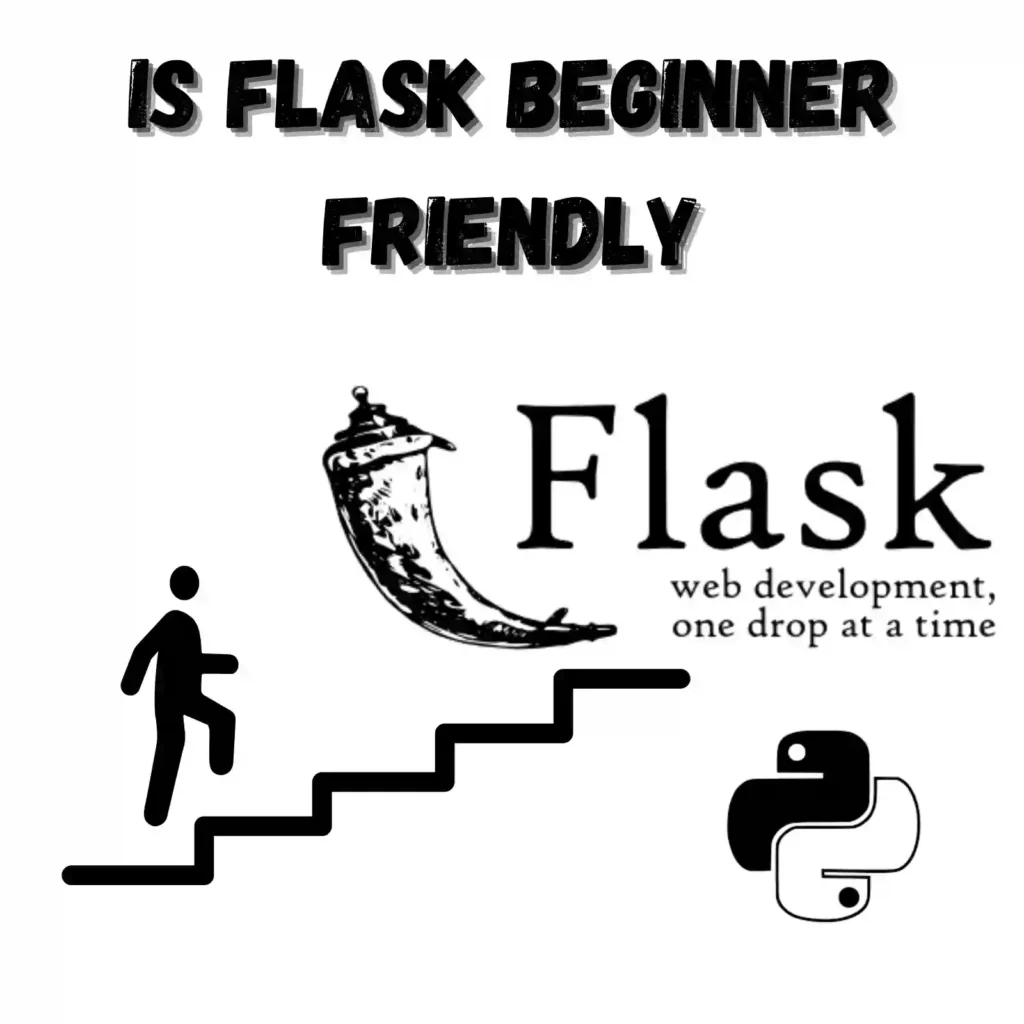
Flask is one of those frameworks that I have used extensively over the last few years. I have grown with it and it has served me well in several projects. The question is though is it friendly for beginners and if so in what areas. The simple answer is that Flask is friendly in pretty much every area you want to use it in ranging from having pre-built minimalism on the code, easy routing, pre-bundled security and a large community that has a lot to offer.
Below I’m going to break down my analysis in different sections so feel free to jump in any of them to get a feel of what’s happening. I’m also going to give you a summary in order to let you decide with a glance if you are looking for an easy answer without too much technical analysis and code examples.
Summary
As mentioned earlier I promised an easy to follow summary of the areas flask is good for beginners. If you are now starting out and trying to decide if it’s a good choice for you and do not want to spend a lot of time researching I have the answers for you in the table below. If for any reason you’d like to know more about any of the categories I list you can check my table of contents above and jump right into the category of interest to you.
Flask | |
---|---|
Minimalism | Great |
Security | Great, pre-bundled |
Community | Very good |
Learning Curve | Easy |
Routing | Good |
Templates | Simple |
ORM | Good (SQLAlchemy) |
Extensions | Average |
From the table above it should be evident that Flask is a great choice for beginners. It has everything you need to cover your Web API needs. I think if you are looking for a quick start minimalistic option it’s unparalleled. If you want a more full blown framework and don’t mind spending time to learn it you can also check out Django which I have a bunch of articles on here.
Minimalism
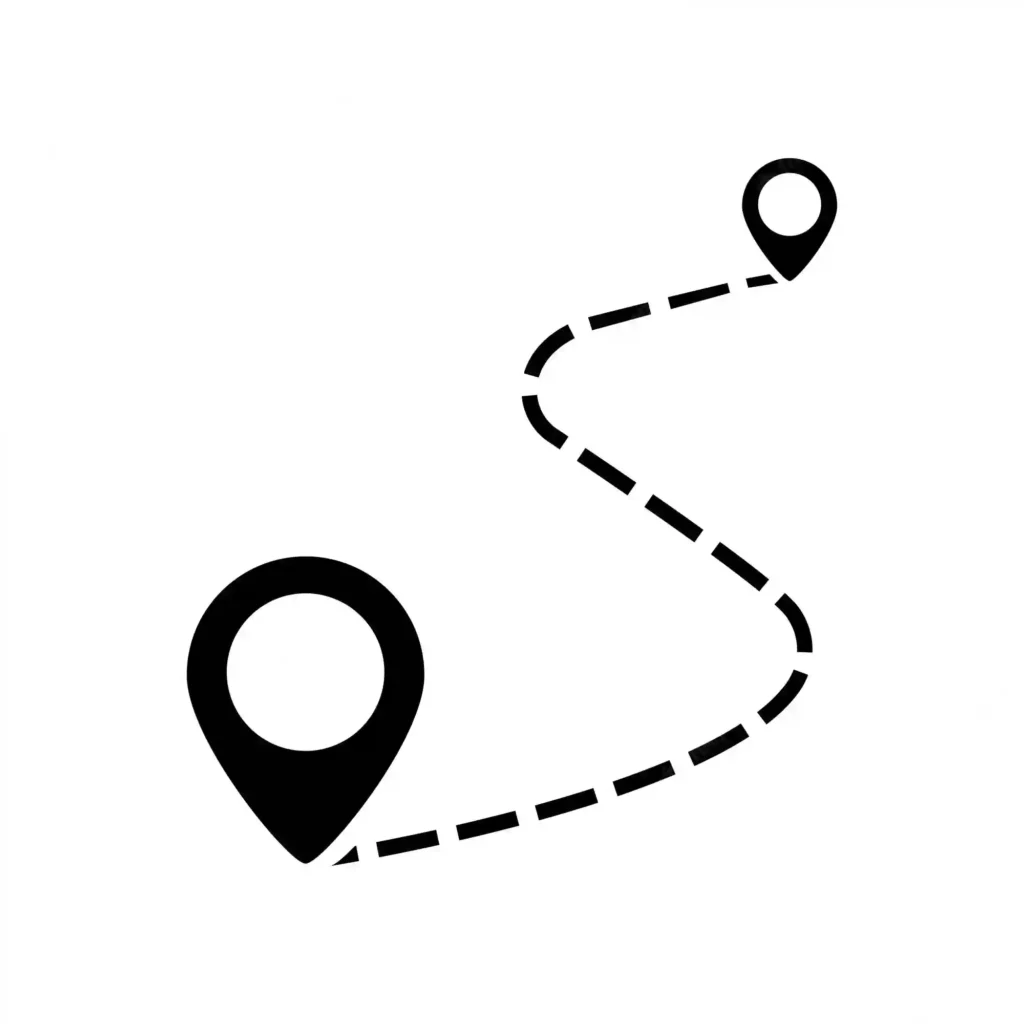
Flask is a lightweight and minimalistic framework, which means it has very few dependencies and is easy to use. It’s designed to be a “micro-framework,” which means it provides only the bare essentials for building a web application. This makes it an excellent choice for beginners who are just starting with web development.
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!'
In this example, we import the Flask module and create a new Flask instance. We then define a function to handle requests to the root URL (‘/’). When a user visits the root URL, the function returns the string “Hello, World!”.
Routing
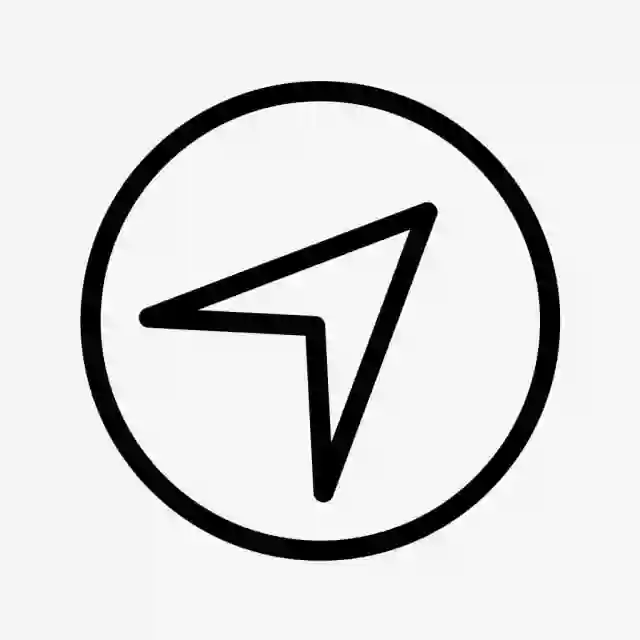
Flask provides an easy-to-use routing system, which allows you to define how URLs are mapped to functions. You can use routing to handle different requests for different URLs. This feature makes it easy to build web applications with multiple pages.
@app.route('/about') def about(): return 'This is the about page'
In this example, we define a new function to handle requests to the URL ‘/about’. When a user visits this URL, the function returns the string “This is the about page”. As you can see adding a route in your app is a breeze and you can get going very fast with it.
Templates
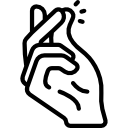
Flask comes with a built-in template engine called Jinja2, which allows you to build dynamic HTML pages with placeholders for content. This makes it easy to build web pages that display data from a database or other source.
<!doctype html> <html> <head> <title>{{ title }}</title> </head> <body> <h1>{{ heading }}</h1> <p>{{ content }}</p> </body> </html>
In this example, we define a simple HTML template with placeholders for the title, heading, and content of the page. We can use Flask’s built-in template engine to fill in these placeholders with dynamic data.
Integrated Development Server
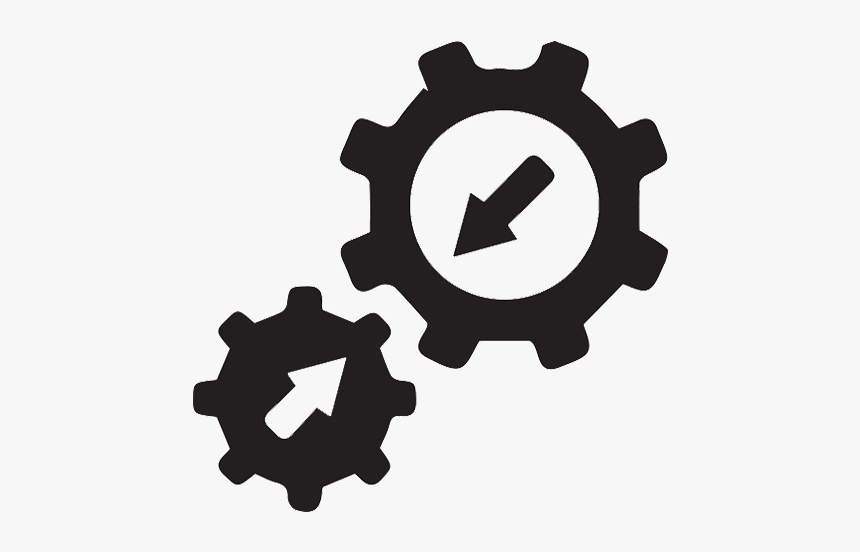
Flask comes with a built-in development server, which allows you to test your web application locally before deploying it to a production server. This makes it easy to get started with web development without needing to install additional software.
if __name__ == '__main__': app.run(debug=True)
As shown above we use a conditional statement to only start the development server if the Python script is being run directly (rather than being imported as a module). We also enable debugging mode, which allows us to see detailed error messages if something goes wrong. One thing I want to stress out here is that by default the debugging and development options for flask are fairly limited if you want to make them better you should look into more extensible options such as Flask debug toolbar.
Flask Extensions
Flask has a large number of third-party extensions available, which can be used to add additional functionality to your web application. There are extensions available for database integration, user authentication, email support, and much more. These extensions can save a lot of time and effort when building web applications.
from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' db = SQLAlchemy(app) class User(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(80), nullable=False) email = db.Column(db.String(120), unique=True, nullable=False) def __repr__(self): return '<User %r>' % self.name
We basically use the Flask-SQLAlchemy extension to define a User model, which we can use to interact with a database. We define three columns for the user’s ID, name, and email, and also define a repr method for easy debugging.
Large Community
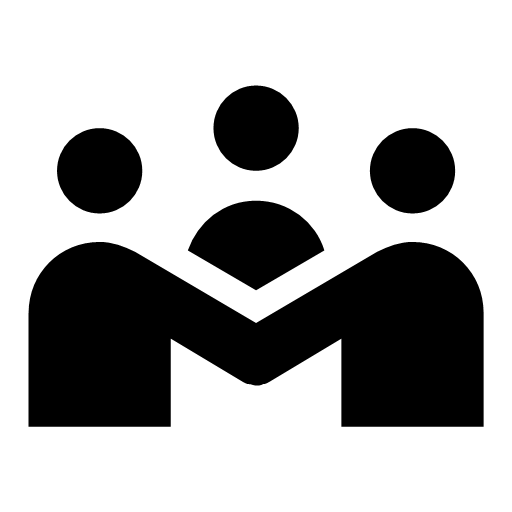
Being a popular framework, Flask has a vast and helpful community. It has numerous forums, online courses, and communities where beginners can interact with experts and learn from their experiences. The community offers excellent support and guidance to beginners who face any difficulty while using Flask. From personal experience every time I had to do a query to find an answer for Flask I got answers fairly quickly. Besides that their documentation is pretty complete which is where a lot of it comes from but also the community puts together a lot of excellent quick start guides that can get you going in no time.
Easy Integration
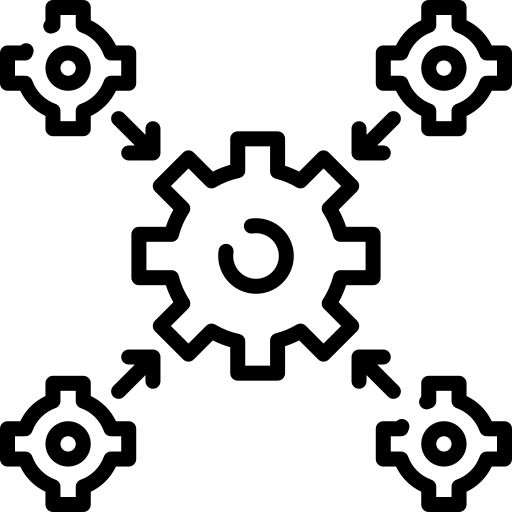
Flask is not only beginner-friendly but also offers an easy and straightforward integration with other technologies. Flask offers simple APIs to connect with popular databases like SQLite, MySQL, and PostgreSQL. It also offers an easy way to integrate with JavaScript frameworks like React, Vue, and Angular.
from flask import Flask, jsonify from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' db = SQLAlchemy(app) class User(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(80), nullable=False) @app.route('/users', methods=['GET']) def get_users(): users = User.query.all() return jsonify([{'name': user.name} for user in users]) if __name__ == '__main__': app.run()
In the code above you can see how easy it is to introduce the SQL alchemy ORM to basically create a data model for your project.
Lightweight and Flexible
Flask is a lightweight and flexible framework, making it a preferred choice among beginners. It does not force you to use any specific tool or directory structure, allowing you to structure your code and files as per your needs. Flask is a perfect fit for building small applications or APIs.
from flask import Flask, request app = Flask(__name__) @app.route('/greet', methods=['GET']) def greet(): name = request.args.get('name') return f'Hello, {name}!' if __name__ == '__main__': app.run()
As we went over some examples before on the flexibility of using modules and python packages such as the requests library it’s pretty lightweight because it doesn’t import a lot of things in the code like other heavier frameworks. This makes it ideal for micro-service driven architectures which could enable you to make a lot of small little Flask instances serving single purpose requests.
Easy ORM Integration
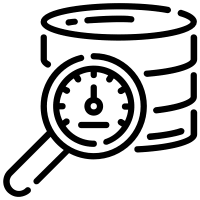
Since I mentioned the ORM earlier I want to show you how easy is to do a more advanced example such as creating an actual model with it. It makes it easy to handle ORMs (Object-Relational Mappers) by allowing developers to choose the ORM that best fits their needs.
from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' db = SQLAlchemy(app) class User(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(80)) email = db.Column(db.String(120), unique=True) def __init__(self, name, email): self.name = name self.email = email @app.route('/') def index(): user = User.query.filter_by(name='John Doe').first() return 'Hello, {}! Your email is {}.'.format(user.name, user.email)
In this example, we first create a Flask app instance and configure it to use an SQLite database. We then create a db
object using the SQLAlchemy
extension.
Next, we define a User
model using SQLAlchemy’s declarative syntax. The model has three columns: id
, name
, and email
. We also define an __init__
method to set the name
and email
attributes. We then create a route to handle requests to the root URL. Inside the route, we use the User.query
object to query the database for a user with the name “John Doe”. We then return a message using the user’s name and email.
Out Of The Box Secure
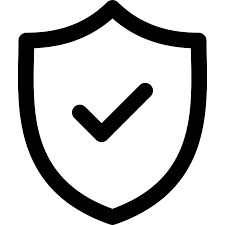
Flask makes it easy to implement security measures in web applications without the need for extensive configuration or additional tools. Here are a few reasons why Flask is well-suited for security:
- Built-in support for secure cookies: It provides a secure cookie implementation that encrypts cookie data with a secret key. This prevents attackers from modifying cookie data or impersonating users.
- Easy to use decorators for access control: Flask’s
@login_required
and@permission_required
decorators make it easy to require authentication or authorization for certain views. These decorators can be easily customized to suit the specific needs of the application. - CSRF protection: It provides built-in support for CSRF (Cross-Site Request Forgery) protection. This is accomplished by including a CSRF token in all POST requests, which is verified on the server side before processing the request.
- Security extensions: Flask has a number of security extensions that can be easily added to an application to provide additional security features. For example, the Flask-SeaSurf extension provides additional CSRF protection, and the Flask-Talisman extension provides secure headers to prevent certain types of attacks.
- Low-level control: It gives developers low-level control over the application, which can be useful for implementing security measures that are specific to the application. For example, Flask allows developers to directly control the HTTP response headers, which can be used to prevent certain types of attacks.
Typically in the past you would have to do a lot of those things manually to keep your code secure, as a beginner this is not ideal as there’s a huge learning curve on how to protect against the attacks we listed above. Flask takes care of this for you without having to do anything!
Cloud and AWS
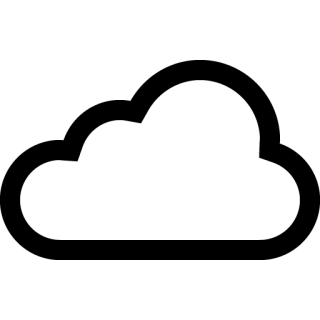
Flask is a good framework for cloud-based applications. As we discussed earlier it has a low overhead and can be easily integrated with other cloud services, such as databases, object storage, message queues, and more. Flask also supports different deployment options, such as deploying to cloud platforms like Heroku, Google Cloud Platform, Amazon Web Services (AWS), and Microsoft Azure. The modular design and extensive documentation make it easy to customize and scale applications for cloud-based environments.
Below I’m going to implement a simple Flask application deployed on AWS Elastic Beanstalk, which is a managed service for deploying and scaling web applications:
from flask import Flask app = Flask(__name__) @app.route('/micro-task') def process_task(): print ('Task is being started now') # execute code here... print ('Task has completed') if __name__ == '__main__': app.run(debug=True)
In this example, we first import the Flask module and create an instance of the Flask class called app
. We then define a route for the application’s home page, which executes any micro task we want it to run in the cloud.
We check if the script is being run directly (as opposed to being imported as a module), and if so, we start the Flask development server with the run()
method.
To deploy this application on AWS Elastic Beanstalk, we can create a ZIP file containing the application code and any required dependencies, and then upload it to the Elastic Beanstalk console. Alternatively, we can use the AWS CLI to create and deploy the application directly from our local machine.
Once the application is deployed, Elastic Beanstalk automatically provisions and manages the underlying infrastructure (such as EC2 instances and load balancers) to handle incoming traffic, and provides features for monitoring and scaling the application as needed.
This is as beginner as it can get making Flask even for the cloud very friendly for people starting out.
FAQs
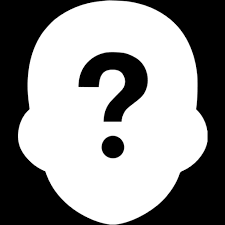
Is Flask only for beginners?
No, Flask is not only for beginners. It is also used by experienced developers to build web applications, APIs, and microservices. Flask’s flexibility and simplicity make it a preferred choice for small projects and prototypes.
How is Flask different from other web frameworks?
Flask is different from other web frameworks in terms of its simplicity, flexibility, and lightweight. Unlike other frameworks, Flask does not force a specific directory structure, database schema, or ORM. It allows you to choose and integrate the tools that you want to use.
Can Flask be used for building large web applications?
Yes, Flask can be used for building large web applications. However, as the application grows, it may become difficult to manage and maintain. For large-scale projects, it is recommended to use a more feature-rich framework like Django or Pyramid.
Is Flask suitable for building RESTful APIs?
Yes, Flask is suitable for building RESTful APIs. It provides simple and intuitive APIs for handling HTTP requests and responses. It also offers easy integration with popular data formats like JSON and XML.
Can I use Flask for building real-time web applications?
Yes, Flask can be used for building real-time web applications. However, it may require additional tools and technologies like WebSockets or Server-Sent Events to achieve real-time functionality.
Related
- Python Flask Multithreading
- How To Stress Test REST API Using Python
- Easy programming languages for beginners