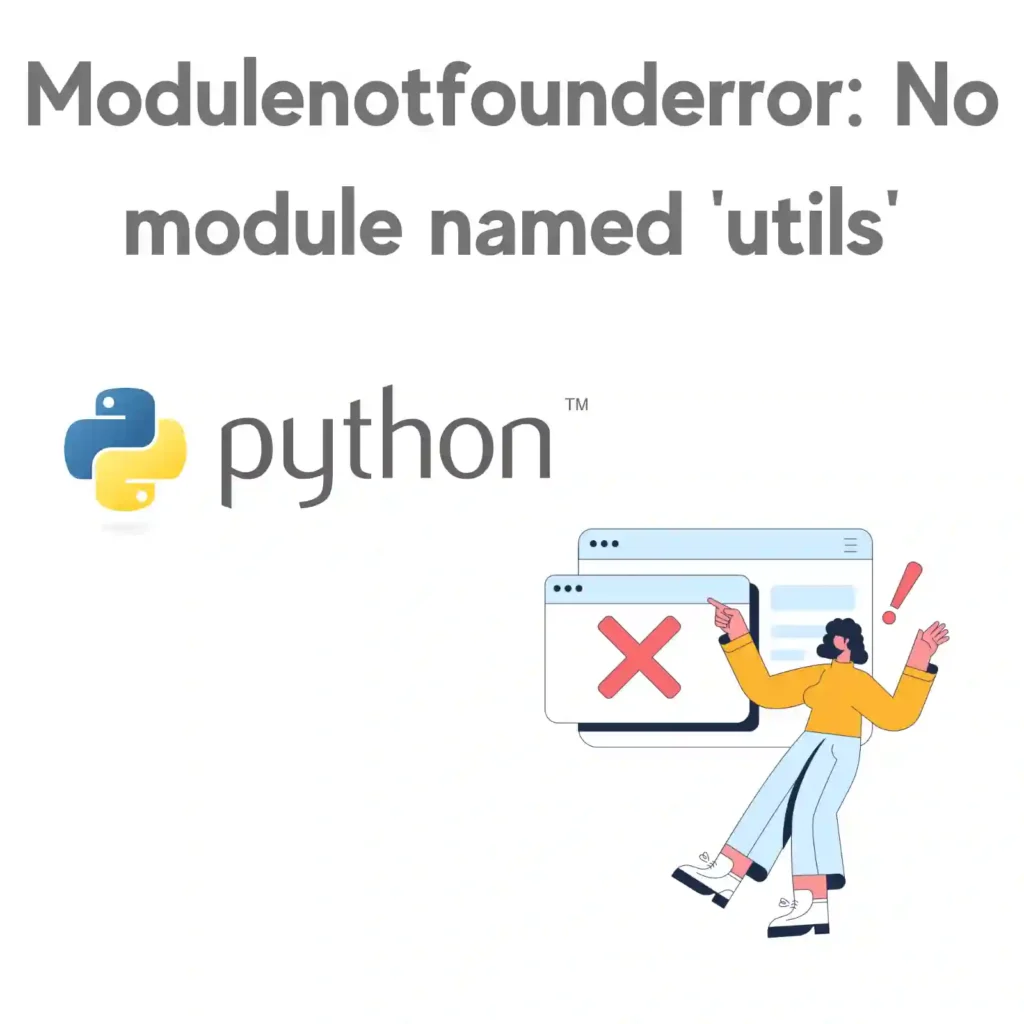
One of the most common problems in Python is the frustrating error message “modulenotfounderror: no module named ‘utils'” at some point in your programming journey. This error message usually pops up when Python cannot locate the module named ‘utils’ that you are trying to import in your code.
In this article, we will explore what causes this error message and provide practical solutions to help you overcome it. We will also discuss how to avoid the error message in the future, ensuring your Python code runs smoothly without any unexpected hiccups.
What is ‘Modulenotfounderror: No module named ‘utils’
Python developers use modules to extend the functionality of their code, and these modules can be imported into their programs as needed.
However, if Python cannot find the module that you are trying to import, it will throw the error message “modulenotfounderror: no module named ‘utils’.” This error message means that Python cannot locate the ‘utils’ module that you are trying to import, and therefore, your program cannot run. Since this can be caused by various reasons below we will break down what may have caused it and how to fix it.
What causes ‘Modulenotfounderror: No module named ‘utils’
There are several reasons why Python may not be able to find the ‘utils’ module that you are trying to import. Some of the most common causes include:
- Incorrect module name: If you misspell the module name or use the wrong case when importing the module, Python will not be able to find it.
- Missing module: If the ‘utils’ module is not installed on your computer, Python will not be able to locate it. You may need to install the module using a package manager like pip.
- Incorrect path: If the ‘utils’ module is located in a different directory than the one you are running your program from, Python will not be able to find it.
- Virtual environment issues: If you are using a virtual environment to manage your Python dependencies, make sure that the ‘utils’ module is installed within the virtual environment.
- Module not installed: One of the most common reasons for this error is that the required module, in this case, “utils,” is not installed. This can happen if the module was not installed properly or if it was not included in the requirements.txt file.
- Incorrect import statement: Another reason for this error could be an incorrect import statement. If the import statement is not properly formatted, Python will not be able to find the required module and will throw the “Modulenotfounderror” error.
- Incorrect module name: It is also possible that the name of the module that the program is trying to import is incorrect. This can happen if the developer misspells the module name or if they are using an outdated version of the module that has been renamed.
- Incompatible versions: The “Modulenotfounderror” error can also occur if the version of the module that is being used is not compatible with the version of Python that is being used. This can happen if the module was developed for an older version of Python and is not compatible with the current version.
- Path issue: If the path to the module is not set correctly, Python will not be able to locate the module and will throw the “Modulenotfounderror” error. This can happen if the module is located in a different directory or if the path to the module is not included in the PYTHONPATH environment variable.
How to fix ‘Modulenotfounderror: No module named ‘utils’
Now that we have identified some of the common causes of the “modulenotfounderror: no module named ‘utils'” error message, let’s explore some practical solutions to help you overcome it.
- Double-check the module name: Make sure that you have spelled the module name correctly and that you are using the correct case when importing it.
- Install the missing module: If the ‘utils’ module is not installed on your computer, use a package manager like pip to install it. You can install the module by running the following command in your terminal: ‘pip install utils’.
- Add the module to your path: If the ‘utils’ module is located in a different directory than the one you are running your program from, add the directory to your path by running the following command in your terminal: ‘export PYTHONPATH=$PYTHONPATH:/path/to/utils’.
- Check your virtual environment: If you are using a virtual environment, make sure that the ‘utils’ module is installed within the virtual environment. You can activate the virtual environment and install the module by running the following commands in your terminal:
$ source path/to/venv/bin/activate $ pip install utils
- Update your Python version: If none of the above solutions work, try updating your Python version to the latest version
- Fix permissions: Your permissions in the running directory where the modules are stored may be incorrect and not accessible. Fixing the permissions will let Python see the module and will start working again.
- Check compatibility: Make sure that the version of the module that you are using is compatible with the version of Python that you are using. If the module was developed for an older version of Python, it may not be compatible with the current version.
- Check the import statement: Similar to the import mispelling you also need to check the syntax of your import statement. Make sure you are using one of Pythons syntax for it such as import or from clauses as shown below.
import module_name
orfrom module_name import function_name
If you have a clause typo or something similar the import statement will fail.
Modulenotfounderror in Different Python Versions
The last thing I want to cover is how the modulenotfounderror exception appears in Python 2.x and Python 3.x respectively.
The ModuleNotFoundError
exception was introduced in Python 3.6, so it does not exist in Python 2.7.
In Python 2.7, the equivalent exception is ImportError
. When a module cannot be found or imported, an ImportError
is raised.
Lets take a look at Python 2.7 first:
try: import non_existent_module except ImportError as e: print("Caught an ImportError:", e)
Let’s run and see what happens:
Caught an ImportError: No module named non_existent_module
In Python 3, the ImportError
exception is still available, but it has been made more specific. When a module cannot be found, the ModuleNotFoundError
exception is raised instead.
For Python 3 this looks like this:
try: import non_existent_module except ModuleNotFoundError as e: print("Caught a ModuleNotFoundError:", e)
So executing this will yield:
Caught a ModuleNotFoundError: No module named 'non_existent_module'
Note that in Python 3, the error message includes the name of the module that could not be found, whereas in Python 2.7, the error message only includes the name of the module that was not found.