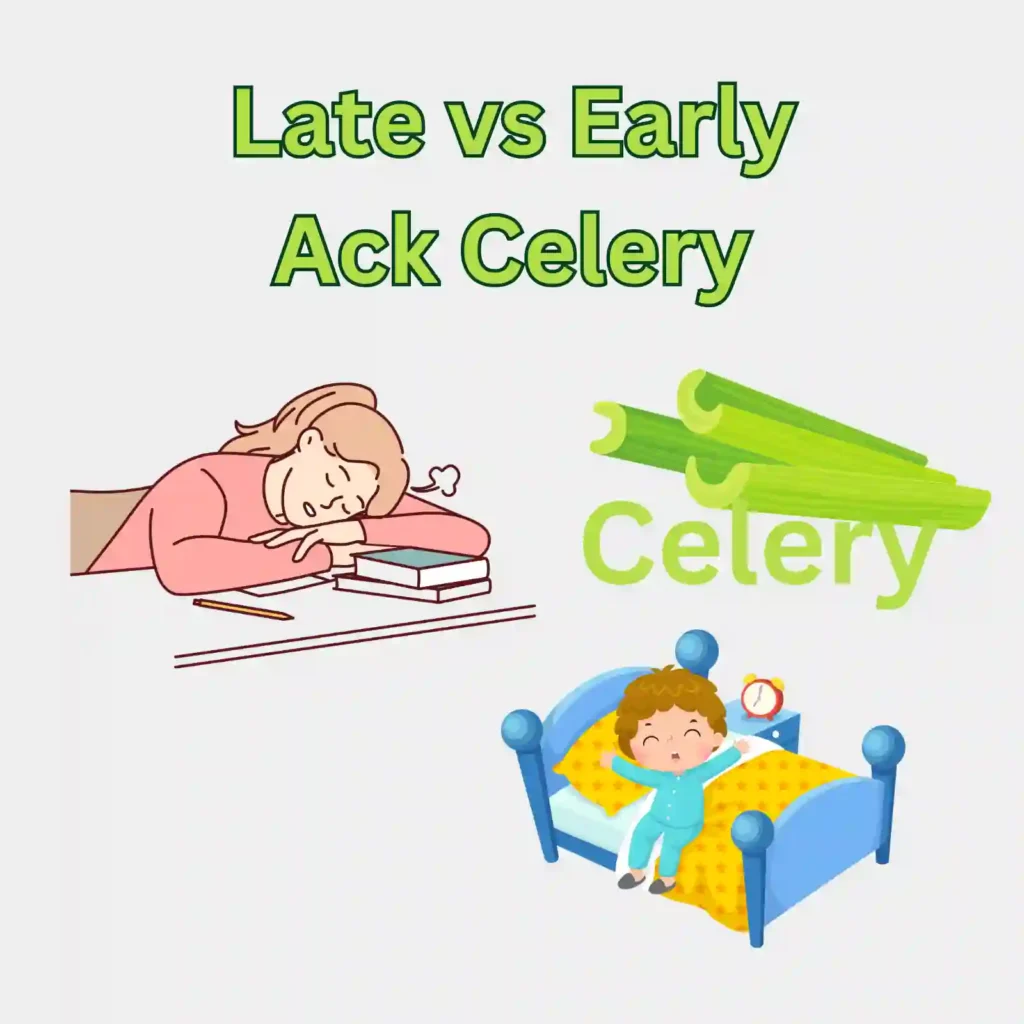
Understanding the difference between early and late acknowledgement in Celery is crucial for efficient task management; early acknowledgement means the task is removed from the queue immediately after consumption, while with late acknowledgement, the task only gets removed post successful execution, thereby optimizing task distribution and error handling.
It’s interesting to delve into the specifics of Celery, a powerful asynchronous task queue/job queue based on distributed message passing. The task acknowledgement feature is pivotal in defining how Celery handles message confirmations. There are essentially two modes, or types, of task acknowledgement: Early Acknowledgement and Late Acknowledgement.
Summary Table
Mode | Definition | Pros | Cons |
---|---|---|---|
Early Acknowledgement (Default) | The task message gets acknowledged by the worker as soon as it receives it, even before starting its execution. |
|
If the worker crashes during a task’s execution, there’s no way for Celery to know the task was never completed because it has already been marked as done. |
Late Acknowledgement | The worker only acknowledges the task message after it completes its execution. | If a worker dies ungracefully while processing a task, the task gets requeued and can be picked up by another worker. | Takes up more broker memory & may lead to message duplication if the worker fails before it acknowledges. |
In considering the implications of early vs late acknowledgement in Celery, it becomes apparent that choice primarily revolves around the trade-off between efficiency and fault-tolerance.
The
'task_acks_late'
configuration option is used to trigger late acknowledgement mode. The default setting is Early Acknowledgement, but this can be overridden at the individual task level if required. If you want Celery to adopt the Late Acknowledgement approach,
from celery import Celery app = Celery() app.conf.task_acks_late = True
This configuration tells Celery to wait until the task has been fully executed before sending an acknowledgement back to the message broker. As noted in the table, this method greatly improves system resilience by ensuring that a task will always get done, even if something happens to a worker during processing.
The trade-offs between these two modes highlight the flexible nature of Celery — the ability to fine-tune your task queuing environment depending upon whether reliability or efficiency is your primary concern. This ultimately underscores the beauty of working with such a versatile tool like Celery in handling asynchronous tasks.
You might find it useful to read more about these settings in [Celery’s official documentation](http://docs.celeryproject.org/en/latest/userguide/tasks.html#Task.acks_late).
Celery is an asynchronous distributed task queue used in Python programming for executing tasks concurrently. Its primary feature of processing tasks asynchronously allows for efficient utilization of system resources, enhancing speed and performance. It’s essential to understand a crucial mechanism within Celery which aids in these tasks—acknowledgements.
The acknowledgement system hinges on the fact that tasks (messages) can either be acknowledged early or late. An “acknowledgement” is basically a confirmation sent by a worker once it receives and starts processing a message. The specific timing of when this acknowledgement is given leads us to two contrasting modes: early acknowledgements and late acknowledgements.
Early Acknowledgements
Early ACKs, as is evident from their name, means that tasks are acknowledged immediately after they’re received, even without completely going through their execution. Once a worker informs the broker about receiving the task, the task is removed from the Queue. As such, there isn’t any way to get the task back if any issue arises during its execution.
# Assigning the acknowledgement mode app.conf.task_acks_late = False
In the code snippet above, setting
task_acks_late
to False initiates Early Acknowledgement.
Late Acknowledgements
On the other hand, late ACKs are where tasks are acknowledged only after their successful execution. In this case, if a worker dies or is restarted while processing a task, the task gets reassigned to another active worker. Thus, Late Ack helps ensure no task goes unprocessed due to complications like worker crashes or sudden restarts:
# Assigning the acknowledgement mode app.conf.task_acks_late=True
In the above code, setting
task_acks_late
to True enacts Late Acknowledgement.
Differences: Early vs Late Acknowledgement
Early Acknowledgement | Late Acknowledgement |
---|---|
Tasks are acknowledged right after receipt, before completion. | Tasks are acknowledged after completion. |
In case of failure during execution, the task cannot be retrieved as it’s already been removed from the queue. | If a task fails halfway for any reason, it is retrieved and re-assigned to another active worker for processing. |
Best suited for scenarios where the relaunch of failed tasks doesn’t impact system performance significantly, or where task failures are less likely to occur. | Preferred for critical tasks where failure can’t be afforded, as it offers a built-in retry mechanism. |
To sum up, the difference between early and late acknowledgement in Celery boils down to when the acknowledgement is sent, and how failure cases are handled. Your choice may differ depending on your task requirements and application needs. More information on Celery and the use of acknowledgements can be found in the official Celery Documentation.
Exploring the Technical Aspects of Early Acknowledgement: Difference Between Early And Late Acknowledgement In Celery
Diving right into our topic, understanding early and late acknowledgement in Celery involves knowing two core conceptual areas; Task acknowledgements and message visibility. Herein, we explore these technical aspects using practical code snippets and concise explanations.
Task Acknowledgements as a concept refers to how your application’s task broker receives confirmation that a worker has successfully received and acknowledged a task’s procession. These confirmations help prevent tasks from getting lost should a worker suddenly stop or crash during execution.
The Python-based distributed task queue system, Celery, offers task acknowledgements through either early or late configuration, which directly affects message visibility.
Let’s put this down in tabular form for clarity:
Acknowledgement Mode | Description |
---|---|
Early Acknowledgement (Default) | The moment a worker receives a task, it sends an acknowledgement to the task broker. This happens before the task execution. |
Late Acknowledgement | In this mode, workers send an acknowledgement only after the task gets fully executed. |
To understand better, let’s see how to implement these different modes in code:
# Setting early acknowledgment (default) app.conf.task_acks_late = False # Setting late acknowledgment app.conf.task_acks_late = True
So when deciding whether to utilize early or late acknowledgements, consider the specific needs and tolerance levels of your Celery application regarding tasks failing or getting lost. If reassurance of receipt is vital, then early acknowledgement seems suitable. However, if task completion is more important, late acknowledgement is the way to go.
Message Visibility is an interrelated concept concerning task acknowledgement. It specifies when a task becomes invisible to other workers once one worker has taken it from the queue.
Remember:
- With early acknowledgement, the task becomes immediately invisible due to the instant acknowledgement.
- With late acknowledgement, the task remains visible until full execution, reducing the risk of losing less critical tasks if a worker goes down mid-execution.
You can also see how these configurations affect scalability and performance. Studies like the one done by ACM reveal scalable architectures benefit from late acknowledgements due to its resilience to failures.
This idea of “acknowledgement” may also draw parallels to the “commitment” concept in database transactions, where transactions get acknowledged- hence, there’s a trade-off between assurance of data durability (akin to task execution in our case) against system performance.
The differences between early and late acknowledgements in Celery, an asynchronous task queue/job queue in Python, can impact application functionality significantly. The Celery system relies on these acknowledgments to verify a worker has received a task and will start executing it. By understanding the functional implications of late acknowledgment in particular, developers are better equipped to design and optimize their applications.
Early Acknowledgement | Late Acknowledgement |
---|---|
By default, Celery sends an acknowledgment message to the broker as soon as a task gets assigned to a worker. This is known as early acknowledgment, but it has its downsides. | Alternatively, tasks can be set up for late acknowledgment, which implies that Celery sends the acknowledgment message once the task has completed or failed, not immediately after assignment. |
celetry_task_ack_late = False (default setting) |
celery_task_ack_late = True |
Early acknowledgement can lead to lost tasks if a worker dies before it has finished processing the task. Even though the broker already received an acknowledgment, implying the server processed the task, the task would be lost as it hasn’t been completed yet. Therefore, if preserving tasks at all costs is a critical concern, early acknowledgment fails to offer this guarantee.
On the flip side, late acknowledgment presents different challenges. With late acknowledgment, if a worker dies, the broker can reassign the task to another worker, since it has not yet received confirmation that the task has finished. This ensures no task gets dropped. However, the downside is that late acknowledgment can lead to tasks getting executed more than once, especially in scenarios where messages have hard timeouts, hence causing repeated retries in short windows.
Deciding whether to engage the early or late acknowledgment strategy revolves around your project’s specific needs and priorities. If the highest priority is executing tasks without failure, even if it means some tasks might run multiple times, late acknowledgment would be the appropriate approach: celery ensures a steady handoff process from when a task enters the queue to its final completion.
However, if the main concern is avoiding task duplication due to repeated retries caused by worker failures or unexpected issues, the use of early acknowledgment is desirable. This way, you prevent repeating tasks but potentially risk losing tasks upon incidents with worker nodes.
When it comes to optimizing task processing in a distributed system using celery, there are important choices to make when it comes to acknowledgements: early or late.
Acknowledging messages play a crucial role in message-based systems like Celery. The way you choose to acknowledge your tasks (early vs. late) greatly impacts not only the efficiency but also the stability of your system.
Early Acknowledgement
In early acknowledgements, as the name suggests, the worker acknowledges the task as soon as it has been received. This means that even before the worker starts executing the task, the task is marked as acknowledged.
The acknowledgment happening directly after the receive call means that whenever a worker crashes while executing a task, the results can be lost because the task was already signaled as done before it was finished– and thus will not be re-sent to another worker. Here is an example:
@app.task(acks_late=False) def add(x, y): return x + y
With early acknowledgment set to False, Celery will acknowledge the task when it’s received by the worker.
Late Acknowledgement
Conversely, in late acknowledgements, the worker sends an acknowledgement only after it finishes executing the task. This implies that if a worker dies while performing a task, that task can be reassigned to another worker. This feature is important for long-running, error-prone tasks commonly found in distributed systems and default setting in Celery.
Here’s how a late acknowledgment looks:
@app.task(acks_late=True) def add(x, y): return x + y
In this case, as specified with acks_late being True, the worker will send an acknowledgment when the task has been executed.
From an efficiency standpoint, both types have their pros and cons. Early acknowledgements are faster because they offload the task immediately upon receipt without waiting for it to complete. Consequently, the queue moves quicker. However, it may not be the most reliable approach if a task fails due to any kind of worker error or crash.
Late acknowledgements, though slightly more “sluggish” as they wait for task completion, ensure better reliability as tasks in jeopardy are reassigned to other workers. As such, late acknowledgements should be used in cases where task completion is critical.
Essentially, the choice between either comes down to what aspect you need to optimize – speed versus reliability, which varies depending on the specific requirements of your application.
For further confirmation about early vs late acknowledgments, you might want to check Celery Documentation. Just remember to run tests with both situations and analyze which one truly suits your needs.It’s interesting to discuss how early and late acknowledgements in Celery, a distributed task queue, can be harnessed for practical applications in different real-world scenarios. To do this effectively, it is crucial to first grasp the differences between the two.
The primary distinction resides in their implications for message consumption and task execution:
Early Acknowledgement: happens as soon as the worker receives the task from the broker. In other words, the message gets acknowledged prior to the consumption process. While this approach aids swift acknowledgment of tasks, there’s a potential risk of loss of messages should the worker crash before the completion of task execution.
Here’s an example of enabling early acknowledgement setup:
app = Celery(broker='amqp://', backend='amqp://', name='my_tasks') app.conf.update(task_acks_late=False)
Late Acknowledgement: on the flipside, occurs after successful completion of the task by the worker. So as opposed to its counterpart, the task won’t get acknowledged unless it has been appropriately processed. This ensures zero loss of tasks even if the worker goes down during task execution. However, it might lead to possible delay in acknowledging messages, depending on the complexity of given tasks.
Here’s a code snippet on implementing late acknowledgement setup:
app = Celery(broker='amqp://', backend='amqp://', name='my_tasks') app.conf.update(task_acks_late=True)
Depending upon specific projects or tasks at hand, developers could harness these acknowledgment models to build more efficient systems. For instance, when deploying tasks critical to your application’s functionality, which simply cannot be lost due to sudden worker crashes, using Late Acknowledgement would prevent data loss via unprocessed tasks. Conversely, Early Acknowledgement could be ideal for situations where speed outweighs the need for keeping every task intact, such as processing large volumes of data wherein occasional losses are bearable.
To outline broader contexts, let’s look into some tables emphasizing real-world examples:
Scenario | Preferred Acknowledgement Model |
---|---|
E-commerce order processing system | Late Acknowledgement |
Data analytics pipeline handling large datasets | Early Acknowledgement |
Email notifications service | Late Acknowledgement |
Location tracking service | Early Acknowledgement |
This table is, of course, simplistic as choosing the right model involves many influencing factors such as task criticality, permissible message loss, and overall system performance requirements. Nevertheless, understanding Early and Late Acknowledgements’ respective implications allows us to optimize our use of the Celery task queue better. It also equips us with strategies for configuring individual tasks or entire celery application instances based on the nitty-gritty of various production environments.
Lastly, it’s recommended to make and assess informed decisions experimentally around which acknowledgment strategy works best using thorough testing and robust logging mechanisms that suit your application instead of blindly picking one over another.When it comes to task processing in Celery, understanding the difference between early and late acknowledgements can significantly shape an application’s behavior and performance.
Early Acknowledgement
{ "CELERY_ACKS_LATE": "False" }
Let’s decode this a bit. With early acknowledgement (CElERY_ACKS_LATE as False), as soon as Celery receives a task message, it sends back an acknowledgment to the message broker. The broker then discards the message from the queue. This behavioural attribute ensures that the number of messages clogging up the broker is minimized. However, it does have its drawbacks. If for any reason, the worker process crashes mid-task, this unrecoverable scenario results because the backup task message has already been discarded by the broker.
Late Acknowledgement
{ "CELERY_ACKS_LATE": "True" }
With late acknowledgement (CElERY_ACKS_LATE as True), on the contrary, task acknowledgments are sent not when they are received, but only after they have been processed. This mode intends to secure against unforeseen worker crashes or failures. In case a worker goes down while performing a task, the broker still holds onto the unacknowledged task message. As such, recovery can be facilitated by reassigning the task to another available worker.
In contrasting both modes:
 | Early Acknowledgement | Late Acknowledgement |
---|---|---|
Acknowledgment Time | After message receipt | After message processed |
Pros | Keeps broker queue lean | Reduces risk of lost tasks |
Cons | Risks unrecoverable tasks on worker crash | Can fill up broker if tasks build up without being acknowledged |
Deciding which approach works best is very much dependent on your specific application needs and constraints. While early acknowledgment keeps your queue clean and efficient, late acknowledgment secures against abrupt, unexpected errors.
Relevant references:Celery Late AcknowledgementCelery Internals: Messages