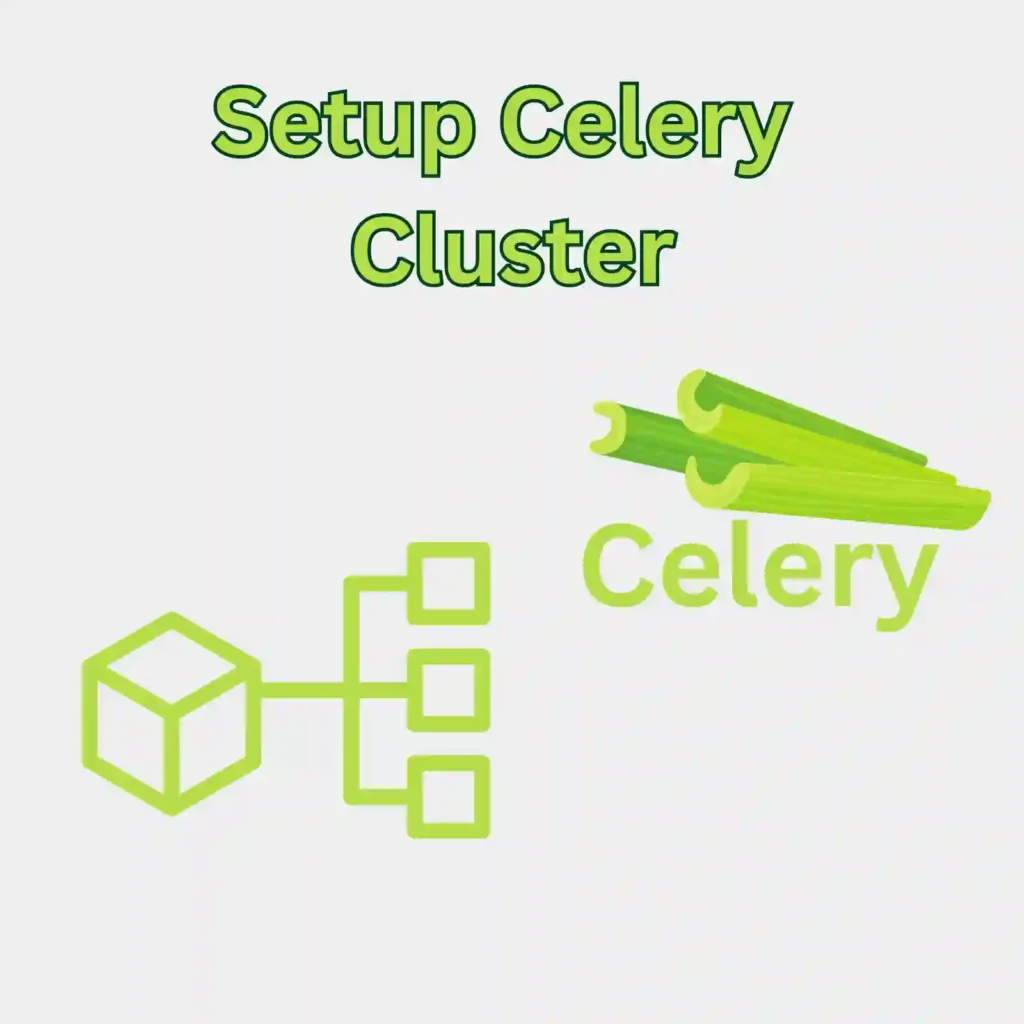
Discover the efficient methods on how to setup Celery in a cluster, optimizing your multi-task operation by enhancing parallel execution and boosting productivity with this reliable distributed task queue system.
Summary Table
Steps | Description |
---|---|
Install and Configure RabbitMQ | To set up Celery for your cluster, begin by installing RabbitMQ as it acts as a message broker. It’s stable, reliable, and allows communication between the main server and worker nodes. |
Install Celery | Next, you need to install Celery on all nodes in the cluster using pip:
pip install celery . This will be used for scheduling and executing tasks. |
Create Celery Tasks | Then, create a Celery instance in your Python code and define the tasks that you want to distribute across the cluster. |
Start Worker Nodes | In each node, run
celery -A your_project_name worker --loglevel=info to start the nodes’ work. |
Invoke Tasks | In the main server, invoke tasks using the method
apply_async() . |
Monitor the Cluster | Finally, this is an essential step that ensures the cluster runs smoothly. This can either be done manually or through web-based tools like Flower. |
Setting up Celery in a cluster invigorates parallel computing which results in faster execution of complex tasks. To do so, one needs carefully managed steps, majorly beginning with installing and configuring RabbitMQ(source). RabbitMQ serves as the message queue necessary for worker nodes and the main server, allowing task dispatching via messages. Then follow the installation of Celery on all nodes involved in the computing.
Creating Celery instances encapsulates defining the tasks to be executed. The command-line interface then fires up these worker nodes, triggering every node to listen for incoming tasks. Tasks, once laid out, remain dormant until invoked—for example, with methods such as
apply_async()
Monitoring the health and productivity of the cluster concludes the setup process. Web-based tools (like Flower) enable real-time tracking and maintenance of tasks. This detailed inspection comprises checking task progress—failed, completed, or running—information about worker nodes, and registering tasks. Thus, setting up Celery in a cluster is a strategically phased procedure that, when combined with effective monitoring, ensures accelerated task execution with minimal downtime.The first step to understanding how Celery functions in a clustered set up is delineating what Celery precisely is. A task processing library for Python, Celery facilitates the asynchronous execution of tasks. In its essence, it’s a distributed system that schedules jobs and processes them in the background.
In a simple environment, you might have one machine executing these tasks. But when you scale up, only running on a single machine may not be feasible. This scenario is where a clustered Celery setup comes in handy.
At its core, a clustered Celery environment involves multiple worker nodes (machines) working synchronously to process the tasks from a shared task queue. As the size of the data and the computational capacity requirements increase, you simply add more machines to your cluster, increasing capacity.
This clustered set-up increases redundancy as well — if one node fails, there are other nodes ready to pick up the slack and continue processing.
To make this happen, we need:
- A message broker (like RabbitMQ or Redis) – Celery communicates via messages. These are usually JSON or pickle formatted instructions.
- A result backend (optional, but recommended) – While not strictly necessary, it’s good practice to keep track of your tasks’ results.
- And of course, Celery itself.
Setting up Celery in a cluster involves essentially the same steps as installing it onto a single machine; you just repeat the process across numerous machines.
1. Set up the message broker
For example, if we choose RabbitMQ as the broker, you can install it by using:
sudo apt-get install rabbitmq-server
Then, activate the RabbitMQ server:
sudo systemctl enable rabbitmq-server
2. Install Celery
Run command to install Celery:
pip install celery
Repeat these two steps across all machines that you want to include in your Celery Cluster.
3. Set up your Celery App
In python source file, setting up a basic celery application would look something like this:
from celery import Celery app = Celery('tasks', broker='pyamqp://guest@localhost//') @app.task def add(x, y): return x + y
4. Initiate worker nodes
Finally, each machine will execute their celery workers with command such below:
celery -A tasks worker --loglevel=info
This set up allows the tasks to be processed asynchronously, building a powerful system that can handle heavy load and distribute it across the Celery cluster. This provides scalability and redundancy, crucial for large-scale projects.
Remember, experimentation is key. Depending on your specific needs, your mileage may vary, especially concerning which message broker to use or whether to include a result backend. But with a basic understanding of what comprises a Celery cluster and how to set it up, you’re off to a great start.
For more information, consider checking out Celery’s official documentation[source]. It’s an excellent resource with in-depth discussion on everything from getting started to complex topics like monitoring and prioritising tasks.In the grand scheme of things, coding and handling intensive web traffic is no easy task. In light of this, Celery comes to our rescue. Celery is a robust asynchronous task queue/jobs queue based on distributed message passing. It aids in distributing work across threads or machines while also allowing you to handle everything from real-time processing to scheduling.
Here’s how you can set up Celery in a cluster:
Installation
The first step is always installation. You’ll need to install Celery via pip:
pip install celery
Setup your Celery application
After installing Celery, you’ll need to create an instance of the Celery library. Let me show you how:
# tasks.py from celery import Celery app = Celery('tasks', broker='pyamqp://guest@localhost//')
In the code above, ‘tasks’ is the name of the current module. Also note that we’ve used RabbitMQ as our message broker.’pyamqp://guest@localhost//’ is the URL to connect to our RabbitMQ instance.
Creating Tasks
Tasks are the building blocks of Celery applications. They encapsulate the work that needs to be executed asynchronously. Here is a simple example of a task that adds two numbers:
# Add in tasks.py file @app.task def add(x, y): return x + y
Running the worker server
To process the tasks in the queue, we need to start a Celery worker. You can do so by using the ‘celery worker’ command as follows:
celery -A tasks worker --loglevel=info
Configuration with Django
If your project is based on Django, you will have to make certain adjustments to ensure Celery works well within the environment. Add the following configurations in your settings module:
# settings.py CELERY_BROKER_URL = 'pyamqp://' CELERY_RESULT_BACKEND = 'rpc://'
And instantiate Celery in your main Django project file like so:
# __init__.py from .celery import app as celery_app __all__ = ['celery_app']
Integration with Docker Cluster
If you’re working with a Docker cluster, you’ll need to incorporate some extra steps to integrate Celery. Within each worker _dockerfile_, include the following lines:
# _dockerfile_ ENV CELERY_BROKER_URL pyamqp://guest@rabbitmq// CMD ["celery","-A","your_project_name", "worker", "--loglevel=debug"]
Next, build your docker-compose.yml file and define your services. A crucial point to remember is that each service should manage its own container. Consequently, if you want multiple workers, just scale up the workers service:
version: '3' services: rabbitmq: image: "rabbitmq:3-management" web: image: django_your_webapp command: python my_web_app/manage.py runserver 0.0.0.0:8000 volumes: - .:/code ports: - "8000:8000" worker: image: my_worker links: - rabbitmq
Initiating the Docker compose service allows you to check if the setup is correct:
docker-compose up --scale worker=4
With just a few lines of code, you’re now running four instances of your worker. Letting you expand dynamically as the demand arise.
Keep in mind that learning new technologies can sometimes be overwhelming and setting up Celery, particularly in a clustered environment, requires a good grasp of not just Python but also understanding of distributed systems and the interplay between synchronous and asynchronous programming. But don’t fret! With continued practice and interaction with these concepts and tools, mastery is indeed achievable!
See documentation here.One of the things you have to consider when running Celery in a production setting is scalability. Scalability refers to how well your Celery application can handle load increases. It’s something that isn’t immediately noticeable during the development phase but becomes consequential when dealing with real-world scenarios where usage can grow exponentially.
Here are some best practices related to scaling and monitoring your Celery cluster:
Scaling Based on Task Types
In a scenario where different task types exist within the cluster, balancing resources becomes crucial. One way to scale is to use queues to separate tasks by their type or resources requirements. Here’s an example of how to set up a queue specific for a task in Celery:
from celery import Celery app = Celery('my_app', broker='pyamqp://guest@localhost//') @app.task(queue='queue_name') def add(x, y): return x + y
With a segregated queue system, you can dynamically assign workers to handle a specific task based on its resource requirement.
Auto-Scaling Workers
Another approach to consider would be auto-scaling your worker instances. This involves spinning up more worker processes to accommodate peak loads and scaling down when demand drops to conserve resources. You specify the maximum and minimum number of worker processes to operate concurrently in your Celery command initialization like so:
celery -A proj worker --autoscale=100,3
Sizing & Provisioning Instances Correctly
Choose appropriately sized instances for your workers. For CPU-intensive tasks, opt for instances with high compute power. Similarly, for I/O-bound tasks, select instance types that offer faster disk access.
Monitoring Your Cluster
Ensuring visibility over your Celery cluster provides critical insights about its performance and helps detect early signs of potential issues. An effective monitoring setup should cover these key elements:
– Task Monitoring: Keep track of your tasks’ status—whether they have been successfully received, are being processed, have faced an error, or completed as expected.
– Worker Monitoring: Monitor worker nodes for their CPU, memory utilization, and task execution times. Any performance dips could indicate computational bottlenecks that need addressing.
– Queue Monitoring: Be vigilant about your message queue’s backlog of tasks. If tasks back up in the queue without being swiftly consumed, it could indicate a lack of worker resources.
Consider using tools like Flower or Prometheus, with their excellent support for Celery to help with this. And as Celery logs runtime metrics and info, always have a good logging setup configured [source].
With all these considerations in mind, managing a scalable and efficiently monitored Celery cluster can become a lot more achievable. Remember that each application has unique needs, so tailor these best practices to suit the specifics of your Celery applications and overall infrastructure.Setting up Celery in a cluster can occasionally present some challenges, but these can be systematically addressed and resolved.
Tackling Worker Isolation
One of the common issues faced while setting up a Celery in a cluster revolves around worker isolation. When workers are unable to communicate effectively with each other or the main server, it creates an isolation issue.
A remedy for this is using a message broker such as RabbitMQ, which ensures that all tasks are properly distributed among the workers. You can set up RabbitMQ following the steps detailed on the official RabbitMQ download page.
Furthermore, you also need to ensure that your celery configurations have the correct broker url:
CELERY_BROKER_URL = 'amqp://myuser:mypassword@localhost/myvhost'
Remember that the username, password, host, and virtual host in the URL needs to be replaced with the correct details pertaining to your RabbitMQ server.
Managing Synchronization Issues
When working in a distributed system like Celery, there can be synchronization issues between the workers if they run at different paces. This might lead to inconsistent and unwanted results.
To manage this issue, use a centralized storage system or a result backend such as Redis. Here is how you can specify celery configurations for Redis backend:
CELERY_RESULT_BACKEND = 'redis://localhost:6379/0'
You can install and configure Redis by following the instructions given on the official Redis download page. Replace the url with your own redis server url.
Ensuring Scalability
Scalability can turn into a problem if not considered initially when setting up your Celery cluster. In case you need more workers in the future, adding them should not create a bottleneck.
The easiest solution here is to ensure every worker added to the cluster has access to the code base. Infrastructure solutions like Docker can be extremely helpful for setting up identical containers with the entire codebase loaded. Docker’s official get started guide may help you in getting up to speed.
In conclusion, setting up Celery in a cluster carries its own share of challenges. However, they can easily be overcome by utilizing appropriate tools for worker communication, managing synchronization issues, and ensuring scaling capabilities. Remember, the key is to ensure that your setup promotes efficient communication, effective synchronization, and ease of scalability. If these three factors are kept in check, your clustered Celery setup will function optimally.
Setting up Celery in a cluster requires a deep understanding of several advanced features like task routing, prioritizing tasks, late acknowledgement, and maintaining high availability on a massive scale. This setup enables the processing of large amounts of data and ensures that your application can handle numerous simultaneous requests without lag.
Task Routing
Having a diverse range of tasks might necessitate the customization of which tasks get executed on which workers. This is where the Celery’s ability to route tasks becomes useful. To ensure that your specially designed tasks are executed by specific workers, you can use routes to guide where tasks should be sent1
celery = Celery('myapp', backend='redis://localhost', broker='pyamqp://') celery.conf.task_routes = { 'myapp.tasks.add': {'queue': 'hipri'}, }
Prioritizing Tasks
Initiating priority for tasks could be instrumental while orchestrating tasks in larger applications or handling important tasks. AMQP based brokers such as RabbitMQ, support priority queueing.2
from celery import Celery app = Celery(broker='pyamqp://', backend='rpc://', broker_transport_options={'queue_order_strategy': 'priority'}) @app.task(priority=9) def add(x, y): return x + y
Late Acknowledgments
This feature tells workers not to acknowledge the task until it’s fully executed. It helps to save resources and ensures all tasks are fully processed. Be careful however, because if the worker crashes or gets reset, the tasks that weren’t acknowledged will be delivered to another consumer3.
@app.task(acks_late=True) def add(x, y): return x + y
High Availability
In a distributed system, one strives to achieve high availability which means minimal downtime. High availability can be achieved by adopting a multi-node setup with dedicated backup nodes like RabbitMQ supports 4. This makes the setup resilient and provides a failover mechanism for when a node is dropped due to hardware failures or network partitions.
The following table presents a summary of the parameters involved:
Feature | Benefits |
---|---|
Task Routing | Controlling and balancing workload between workers |
Prioritizing Tasks | Ensuring crucial tasks get executed first |
Late Acknowledgements | Saving resources and ensuring complete task execution |
High Availability | Minimal downtime and resilience against failure |
These characteristics set the foundation for designing a robust and highly scalable Celery cluster. While guiding through a hands-on setup falls beyond the scope of this piece, complete guidelines are available in their official documentation5.
After understanding the role and importance of Celery in task queuing and asynchronous job execution, we proceeded to discuss the steps involved in setting up Celery in a cluster environment. Starting with the installation of necessary components like RabbitMQ and Redis, we discussed how to create virtual environment using venv and pip, providing isolation and dependency management in your Python project.
We used
celery -A your_project_name worker --loglevel=info
to start the workers and monitored task with flower dashboard. We also highlighted careful configuration settings by appropriately setting concurrency levels, the number of processes/threads per worker, and other options which directly influences the optimum utilization of resources on your nodes.
The example code provided serves as an illustrative guide and can be modified based on specific application needs. It may not only be beneficial for those needing to process data asynchronously but also serve as a starting point for those looking to understand distributed task queues and its implementation.
To aid in further exploration, the official Celery documentation[1] is a rich resource and a must-read to capitalize on all features offered by this powerful tool. For any challenges encountered during setup, the vibrant community of developers around Celery are another valuable resource that you can turn to for problem-solving and best practices.[2]
Key Takeaways |
---|
– Celery is a powerful tool for task queuing and asynchronous job execution |
– Properly configuring Celery in a cluster environment is crucial for efficient utilization of resources |
– The Celery community and official documentation are handy resources for troubleshooting and learning more about this tool. |
Through this, I hope I’ve been able to provide substantial insights on how one can go about setting up Celery in a cluster, while shedding light on some of the benefits of doing so. Happy coding!