Introduction
Today we are going to discuss about 7 Django extensions that will make your life easy in Python 3. As many of you already know Python Django is a framework that brings in Web API capabilities along with rendering pages for Python so it’s a complete solution. This does not mean you need to use both the template frontend generation and the backend part. In this article I’m going to focus mostly on the backend portion and useful extensions that go along with it.
I have been working in the Software industry for over 23 years now and I have been a software architect, manager, developer and engineer. I am a machine learning and crypto enthusiast with emphasis in security. I have experience in various industries such as entertainment, broadcasting, healthcare, security, education, retail and finance.
Python alongside with Django is a very good combination, over the years there were some off the box extensions that make it supercharged and reduce the amount of work you would have to do in terms of coding and debugging. Below I’m going to split the extensions based on the categories I believe are useful and can reduce your workload while introducing some features that make it more manageable.
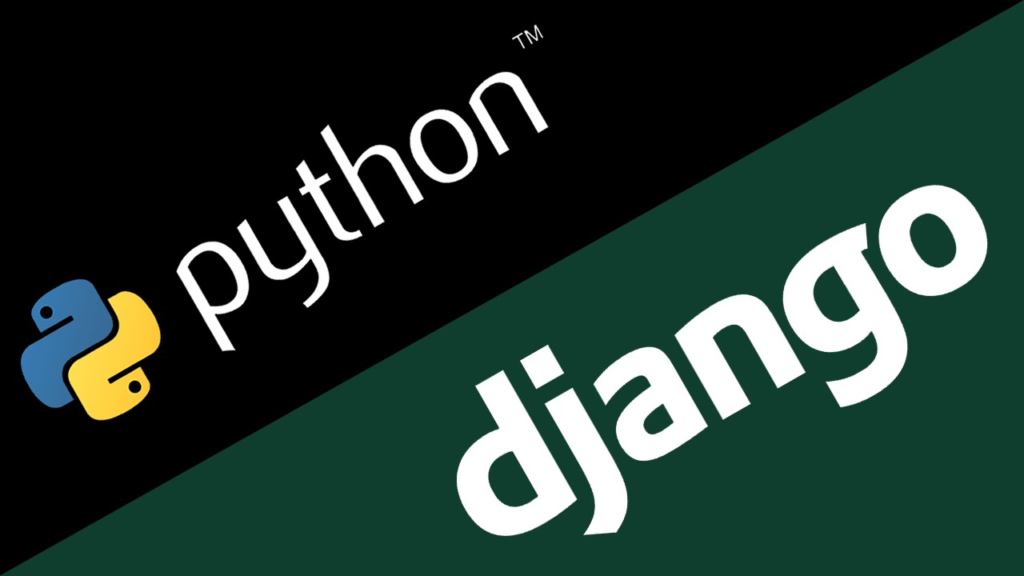
How to add REST functionality in Django
Django Rest Framework
If you are considering of making an application that is based on a REST API this is a must have plugin as it makes everything REST related great for Python Django. Before we go into any details of how this will help you lets outline what REST is and why you should be using it in your application. To summarize this REST is an application interface that sets a standard for frontend and backend developers to use as an entry point for developing complex applications. It relies in 4 pilars on top of the HTTP protocol:
- GET: This is used for retrieving information from the API (can optionally take an ID for specific retrievals)
- POST: This is used for adding a new record into your system
- PUT: This is used to update in place an existing record and it’s usually accompanied by an ID of the record
- DELETE: This is used to remove a record of the system
Once you understand those 4 basic HTTP methods then it’s fairly easy to have a standard way of communicating and bridging the gap between developers. Another important point to note here is that POST and PUT is often misused as to what it does please don’t be the person that uses POST to update a record and PUT to add a new record into the system. Having said that the form of communication typically that is used along with REST frameworks is JSON.
If you are not familiar with the JSON format here’s a snippet of how it looks, it’s basically a bracket based format that takes keypairs of variable name and value an example of a JSON object describing a person could be the following:
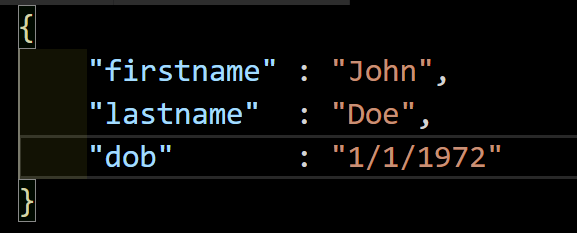
This extension allows you to combine all the powerful features of a REST API and brings it into Django in an abstracted way. It will save you from writing a lot of code and with the help of some powerful functions it has it will allow you to have cleaner and easier to debug code. You can find more about how to do this from scratch in my blog article on how to setup python django rest framework. If you would like to drill into more details on the django-rest-framework documentation you can find the projects page here.
Django Extensions
Another very powerful plugin that offers a magnitude of things is the Django Extensions plugin. To summarize this plugin lets start by breaking it down to different sections below. You may find more information about this plugin in this page.
How to get an extended shell in Django
The Django extensions shell plus item is very useful as it offers an enhanced way of interacting with the models installed in your system. It lets you auto-complete among other things and be able to drill down to the details of an object without having to go back to your code and find it. Also it handles a lot of your automatic imports when it loads up allowing you to save a lot of time by typing things manually.
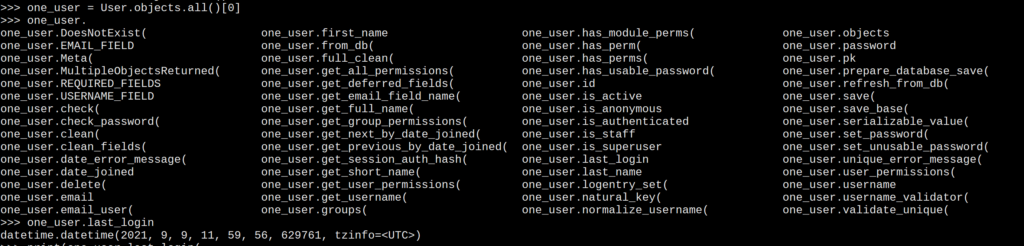
One of the easiest use cases for me is when I’m trying to implement a Django view and I want to quickly experiment with the ORM objects. Since everything is already imported automatically I can immediately start typing in queries in my ipdb debugger and paste them as-is inside my Django view business logic. The time savings just from this are enormous especially considering you are mainly dealing with data in most of your API calls.
How to get an extended runserver in Django
Another useful package is the run server plus. It includes an embedded debugger that leverages an interactive shell like ipdb when things go south and your api view fails. It also has some coloring mode that makes the logs easier to diagnose if there are a lot of API requests. The thing I like the most about it though is being able to get a full traceback and drill down when an exception happens at the point it happens and identify all the issues it has.
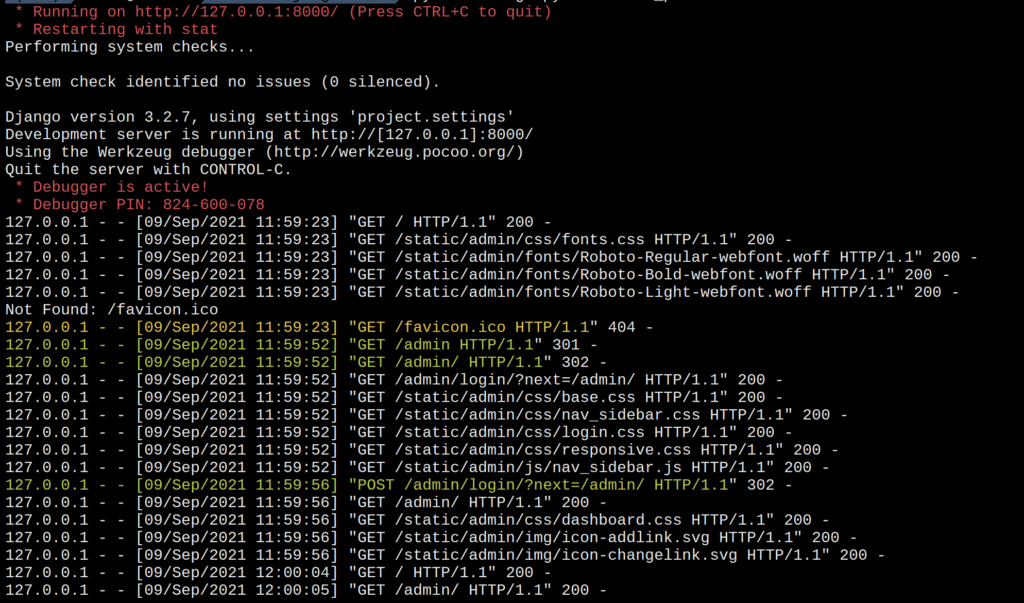
One of my favorite use cases here is when I’m debugging a problem with an app or a web frontend that’s making a lot of API calls. Typically this is hard to read when debugging and you may lose some of the exceptions or failures but there is an option that comes handy here where it can open up your browser directly with the exception if this occurs. Another useful thing about it is that it supports SSL so if you have some specific problems that only happen when SSL is turned on runserver_plus lets you do that and get the bonus of all the debugging capabilities you have with the normal server.
How to run Django scripts
One of the things you may want to do is run a script that interacts with your Django project. In this particular case it could be accessing data models and retrieving information, running a one time database fix-up script, archiving, backup or whatever you may think. If you want to do this outside the API ecosystem and still inherit all your Django settings is with the use of a script. This command is particularly useful when dealing with such tasks. An example of it below is just listing the users of the system.

How to run Django jobs
Another very helpful and similar extension to run script is the ability to run background jobs. This is typically done with something like Celery but django extensions abstracts this and offers a similar approach of running scheduled jobs. The structure is usually split in various options you will have such as:
- Hourly
- Daily
- Monthly
- Weekly
- Yearly
Allowing flexibility to run those long executing tasks that would previously run as a clean-up script, archiving, error checking, dead link checking and a lot of other miscellaneous tasks that can get scheduled. An example below runs a simple hourly job manually invoked from the command line:

I got into extensive detail describing how to setup your environment to run such jobs without having to do much guesswork or read complicated documentation in my Python how to setup background jobs article.
How to find Django settings
Sometimes we are left wondering what is the best way to find all internal settings in a Django application or diagnose what the setting variable after all checks is set too. Print settings solves this problem showing you the final settings Django is using. This is particularly useful if you are diagnosing problems such as identifying the locale or language used, what timezone you are in after all your checks etc. It gives you complete control over your code base as you can invoke this programmatically and do things on the fly as your code runs.
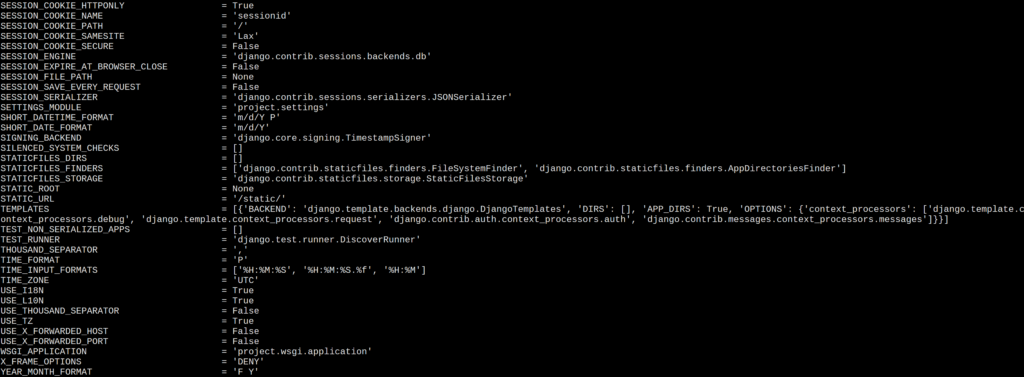
How to Debug Django – Wekzeug
Ok I have to admit this sounds like the weirdest name for a python pip package. I often have to google the name before I type it in pip to install it! Having said that it’s a great plugin!! It offers to you in conjunction with Django Extensions to debug interactively in a shell what exception you are getting. You get a little prompt just like you would with ipdb and can interactively print out environment variables, python variables, check the stack of your execution at a nice visual way which makes fixing issues a breeze. This plugin alone has helped me fix really complicated issues saving hundreds of hours of debugging time and putting print outs in my code to find the root cause.
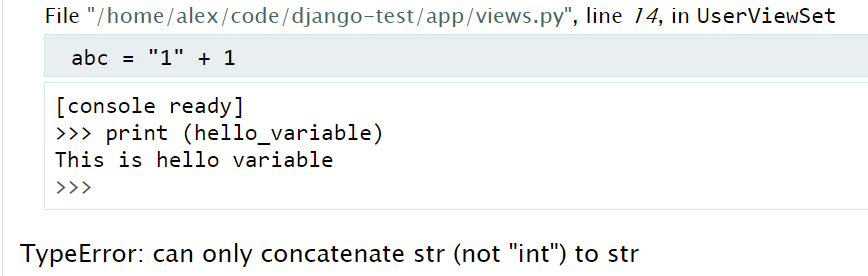
Yes this is correct what you are seeing above is a type error exception I introduced in an API view to see it being captured. Not only that I’m able to use an IPDB shell at the point of the exception and print out anything prior it. Of course the full traceback is also visible and can be seen below.
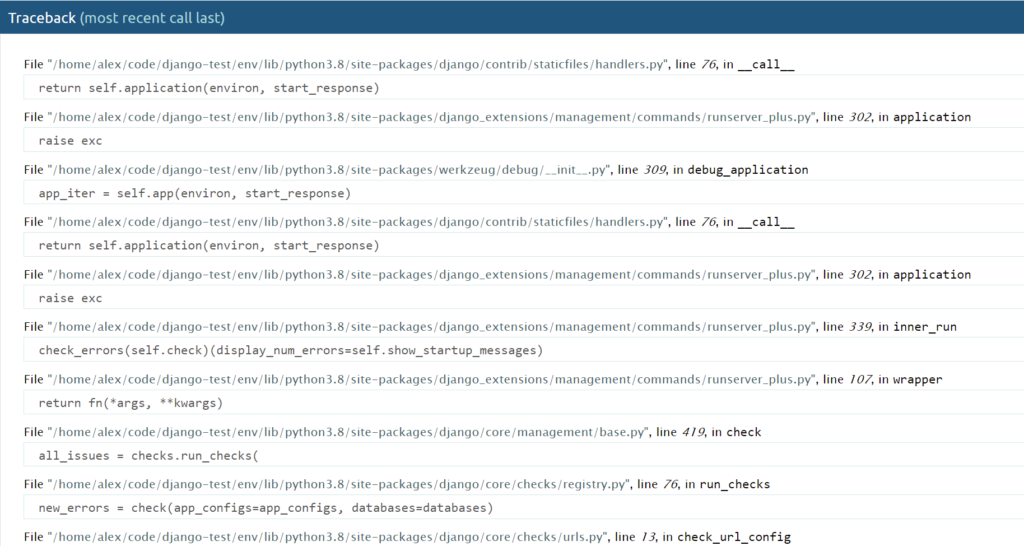
If you want to learn more about how to set this up feel free to read my Python Django debugging article where I walk you through the entire process on how to set this up and use it, along with some small extras on debugging in general that may be helpful for you.
Conclusion
One thing to keep in mind is that Python Django is a very versatile framework which means you can do the same thing in many different ways. I tried to focus on how to do things with avoiding to write too much boilerplate code and have re-useable components that are easy to debug. Hope you found the 7 Django extensions that will make your life easy (Python 3) helpful and you may integrate some of them in your future projects.
If you found this article useful and you think it may have helped you please drop me a comment below I would appreciate it.
Don’t forget to drop me a line for any questions, comments below I check periodically and try to answer them in the priority they come in.
Also if you have any corrections please do let me know and I’ll update the article with new updates or mistakes I did.
If you would like to learn more about Python please take a look at my section here for more articles.
I have also written an extensive guide on the Django Rest Framework setup if you would like to check it out you can find a link here.
Which Python Django extension you find useful?
You can find some relevant Django articles below: