Introduction
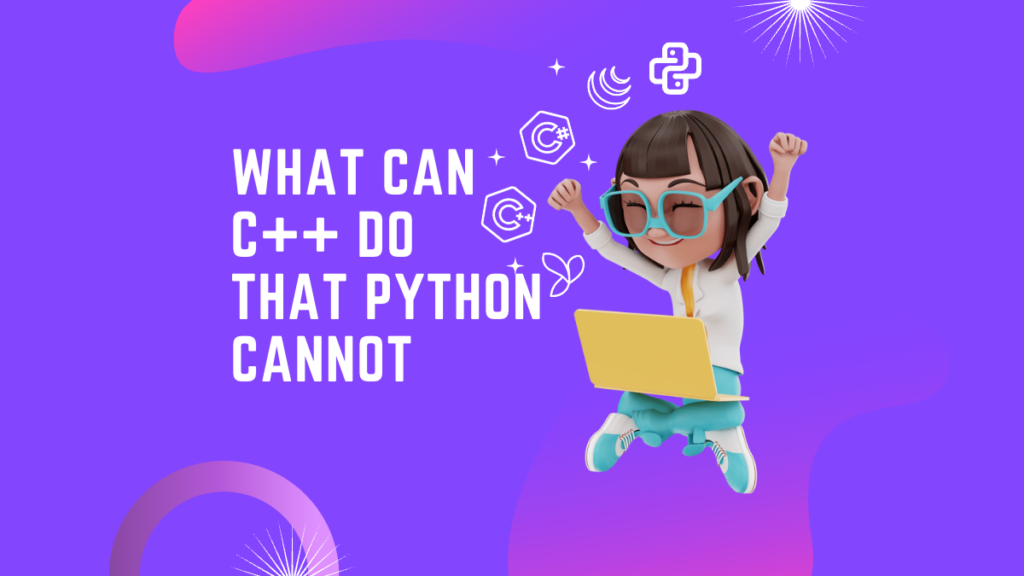
I am always fascinated by the pros and cons of various programming languages particularly when it comes to two of my favorite ones Python and C++. While I enjoy the simplicity and ease-of-use of Python, it would be ignorant to dismiss the strengths of other languages, such as C++. In this blog post, I will discuss some of the capabilities that are exclusive to C++, despite Python’s growing popularity as a versatile language for scientific computing, web development, and more.
In certain situations, C++ might deliver a more robust, higher-performing solution than Python, and it’s important to consider these instances when faced with a challenging programming problem or project. I’ll delve into my personal experience and discuss specific scenarios and capabilities where C++ outshines Python, illuminating the key differences and advantages of both languages.
Quick Comparison
Before we go into an in-depth analysis I’d like to summarize it in a table for those of you that want quick answers and don’t have time to delve into the details of this.
Feature | C++ | Python |
---|---|---|
Manual Memory Management | Allows explicit memory allocation and deallocation | Utilizes automatic memory management (garbage collection) |
Inline Assembly | Provides the ability to write inline assembly code | Lacks direct support for inline assembly |
Operator Overloading | Allows redefining the behavior of operators for user-defined types | Supports operator overloading to a great extent |
Performance Control | Offers fine-grained control over performance optimizations | Optimizations are primarily handled by the interpreter |
Low-level Hardware Interactions | Enables direct interaction with hardware and memory | Lacks low-level hardware access |
Strongly Typed | Employs strong type-checking and static typing | Relies on dynamic typing |
Preprocessor Directives | Utilizes preprocessor directives for conditional compilation and macro expansion | Lacks a preprocessor with similar capabilities |
Multiple Inheritance | Supports multiple inheritance for classes | Only supports single inheritance |
Template Metaprogramming | Allows compile-time code generation and transformation | Lacks a similar level of compile-time code manipulation |
Standard Library | Provides a rich standard library with various functionalities | Offers a comprehensive standard library as well |
Performance and Efficiency
The most prominent advantage of C++ over Python is its high performance, which is mainly attributed to the below factors.
Compilation
C++ is a compiled language, meaning the code is converted to machine language before being executed. This allows for many optimizations through static analyses, leading to faster execution times. On the other hand, Python is an interpreted language, resulting in significantly slower runtime performance due to additional overhead for interpretation.
Inline Assembly
C++ provides the ability to write inline assembly code, allowing direct interaction with the processor and access to hardware-specific features. This can be useful for low-level optimizations or implementing hardware-dependent functionalities. Python, being an interpreted language, lacks direct support for inline assembly. If you take a look at the example below this is evident how you can even use assembly!
#include <iostream> int main() { int value = 10; // Inline assembly to increment the value using the 'add' instruction asm("addl $1, %[value]" : [value] "+r" (value)); std::cout << "New value: " << value << std::endl; return 0; }
Memory management
C++ gives developers more control over memory management, allowing them to optimize memory allocation and deallocation manually. This increases efficiency in situations where performance is critical. Python uses garbage collection to automatically manage memory, causing a slight delay in retrieving or releasing resources.
Manual Memory Management
C++ allows programmers to manually manage memory using features like pointers and explicit memory allocation/deallocation with new
and delete
. This level of control can be beneficial for performance-critical applications and low-level systems programming. Python, on the other hand, utilizes automatic memory management through garbage collection, relieving developers from manual memory management concerns.
Coming from a C background I can tell you for a fact that C++ makes it easier having to deal with malloc/free and keep track of things. Even the best more experienced programmers that implement kernels tend to forget this. Off course this has a big implication too that it may lead to a lot of security problems such as a double-free or memory corruption if the data allocated is not sufficient such as stack or heap overflows.
#include <iostream> int main() { // Dynamically allocate memory for an integer int* ptr = new int; *ptr = 42; std::cout << *ptr << std::endl; // Explicitly deallocate the memory delete ptr; return 0; }
Close-to-metal Programming
C++ can interact with hardware at a lower level than Python, making it ideal for system-level and embedded software development. As an example, C++ enables developers to access memory addresses directly using pointers, providing better control over memory manipulation. Python inherently lacks this feature, relying on abstracting these low-level interactions.
Template Metaprogramming
Template metaprogramming in C++ is a powerful technique that allows developers to write generic and reusable code while generating efficient programs. It involves writing code that gets instantiated during compilation based on templates and type parameters. Although Python has built-in generics through modules like typing, they do not achieve the same level of flexibility and performance.
Operator Overloading
C++ supports operator overloading, allowing developers to redefine the behavior of operators for user-defined types. This provides flexibility and enables intuitive syntax for custom classes. Python has extensive operator overloading capabilities as well, but it does not support low-level customization to the same extent as C++.
#include <iostream> class Complex { private: double real; double imaginary; public: Complex(double r, double i) : real(r), imaginary(i) {} Complex operator+(const Complex& other) const { return Complex(real + other.real, imaginary + other.imaginary); } void print() { std::cout << "Real: " << real << ", Imaginary: " << imaginary << std::endl; } }; int main() { Complex c1(2.5, 3.7); Complex c2(1.3, 4.2); Complex sum = c1 + c2; sum.print(); return 0; }
Multiple Inheritance and Object-Oriented Programming
While Python supports the concept of multiple inheritance, where a class can inherit from more than one base class, C++ offers more versatility when it comes to object-oriented programming. C++ is equipped with access specifiers (public, protected, and private), enabling developers to have finer control over the visibility and usage of class members.
Multi-Threading
Although Python provides support for multi-threading, it suffers from a well-known limitation referred to as the Global Interpreter Lock (GIL). Due to the GIL, only one native thread can execute at a time within a single Python process – even on multi-core processors. This restriction hampers concurrent execution and limits the full utilization of modern CPUs’ capabilities.
On the other hand, C++ natively supports multi-threading without any critical constraints, enabling developers to exploit true parallelism on multi-core processors effectively. As a result, C++ programs can achieve better performance and efficiency when it comes down to tasks that are heavily threaded or parallelizable by nature.
Better Compilation Error Information
The C++ compiler offers richer error messages when code compilation fails. This helps developers detect and fix errors more efficiently compared to Python interpreters, which may sometimes yield vague or generic error messages.
Security
Although Python is fairly secure by default with C++ you can tap into Operating system specific features that are typically hard to access via a higher level language such as Python. For example initially ACL (Access Controls) were not easily modifiable now with the help of libraries you can do this but C++ was always able to do this as it’s a System level language.
Additionally it can benefit with changing memory permissions other than the default parameters to do more security such as having read-only pages and dynamically adjusting them for a write when needed.
Binary Size and Resource Consumption
As mentioned earlier, C++ code is compiled directly into machine language, producing small binary files in turn. Meanwhile, Python applications rely on an interpreter, usually leading to larger package sizes when bundling with the necessary dependencies.
Superior Library Support for Certain Use Cases
Although Python’s standard library and third-party packages offer a comprehensive repository of tools for various applications, C++ excels in specific domains like computer graphics, video game development, and high-performance computing. It benefits from libraries such as OpenGL, DirectX, and Vulkan for rendering graphic content, along with Boost and Parallel STL for concurrent programming.
Deterministic Destructors
In C++, destructors are executed deterministically as the variable goes out of scope, enabling cleaner resource management if an object holds system resources such as file handles or network connections. Conversely, Python relies on garbage collection to reclaim resources, potentially leading to unpredictable destruction order and lag.
Embedded Systems Development
Another area where C++ has an unmistakable edge is in embedded systems development. Embedded systems typically have strict constraints on hardware resources such as processing power, memory, and energy consumption, which necessitates careful consideration when selecting a programming language. Here, C++ is a go-to option owing to its low-level control of memory and hardware, as well as its strong potential for optimization.
Python does have MicroPython and CircuitPython for microcontroller programming, but their use is still limited compared to the ubiquity and maturity of C++ in this sphere. Additionally, Python’s larger memory footprint and slower performance render it less than ideal for many resource-constrained embedded environments.
Game Development and Graphics Rendering
C++ has long been a staple in the realm of game development and graphics rendering, particularly when it comes to high-performance 3D applications and engines. As performance is usually of paramount importance in both these areas, C++’s efficiency and raw power justify its status as an industry standard. Major game engines such as Unreal Engine and Unity (for its high-performance backend) are built on C++.
While Python has libraries like Pygame, Panda3D, and even binding modules for popular engines like Godot, they generally don’t measure up to the prowess of their C++ counterparts in terms of performance. For small-scale, less demanding games Python may be sufficient, but for serious game developers and graphics programmers, C++ remains the go-to choice.
System-Level Programming
System-level or operating system (OS) development is another sphere where C++ reigns supreme due to its ability to directly interact with hardware resources. This capacity to access low-level constructs enables developers to write and manipulate systems software such as OS kernels, drivers, and utilities—tasks that simply wouldn’t be feasible using Python due to its higher level of abstraction and automatic memory management.
Real-Time Applications
When it comes to real-time applications such as robot navigation systems, flight control software, or stock trading algorithms, predictability in performance is critical. These systems require responsiveness in the order of microseconds – a level of precision that necessitates a programming language closely intertwined with the underlying hardware.
C++ is designed to deliver this precision, allowing developers to manage resources and execute operations directly on the hardware with minimal lag. Python’s comparatively slower performance and garbage-collection-based memory management may introduce undesirable delays in real-time scenarios.
Conclusion
While Python is highly valued for its simplicity and readability, there are certain aspects in which C++ surpasses Python’s capabilities. Performance, low-level programming, template metaprogramming, and advanced object-oriented support are areas where C++ has an edge. Nevertheless, selecting the best language for a project depends on various factors, such as the desired outcome, existing experience, and project requirements.
On a personal note, although Python has allowed me to develop projects quickly and efficiently, acknowledging languages’ strengths, like C++, opens up possibilities for creating better and more optimized applications. As developers, we must be open to learning and appreciating different programming paradigms and finding ways to keep expanding our skill sets.
So, bear in mind that there’s no one-size-fits-all answer – instead, always start by understanding your project’s specific needs and select the language that best aligns with those requirements!